mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-13 17:27:20 +00:00
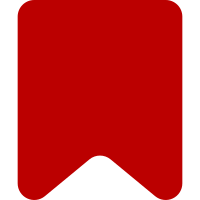
Now it's always wider, and so is the "notes" field. Moreover, the fallback textarea has the exact same size. Plus removed a parameter which only made it hard to write a CSS rule for the textarea. Since the textarea is generated by the same code, and we're always using it for the same thing (filter syntax, regardless of the final goal), make it always use the same name. Bug: T230591 Change-Id: Ibb308e80d954c0e81aa09249c38c39572f157948
116 lines
2.9 KiB
JavaScript
116 lines
2.9 KiB
JavaScript
/**
|
|
* JavaScript for AbuseFilter tools
|
|
*
|
|
* @author John Du Hart
|
|
* @author Marius Hoch <hoo@online.de>
|
|
*/
|
|
|
|
( function () {
|
|
'use strict';
|
|
|
|
/**
|
|
* Submits the expression to be evaluated.
|
|
* @context HTMLElement
|
|
* @param {jQuery.Event} e The event fired when the function is called
|
|
*/
|
|
function doExprSubmit() {
|
|
var expr = $( '#wpFilterRules' ).val(),
|
|
api = new mw.Api();
|
|
$( this ).injectSpinner( { id: 'abusefilter-expr', size: 'large' } );
|
|
|
|
api.post( {
|
|
action: 'abusefilterevalexpression',
|
|
expression: expr
|
|
} )
|
|
.fail( function showError( error, details ) {
|
|
var msg = error === 'http' ? 'abusefilter-http-error' : 'unknown-error';
|
|
$.removeSpinner( 'abusefilter-expr' );
|
|
$( '#mw-abusefilter-expr-result' )
|
|
.text( mw.msg( msg, details.exception ) );
|
|
} )
|
|
.done( function showResult( data ) {
|
|
$.removeSpinner( 'abusefilter-expr' );
|
|
|
|
$( '#mw-abusefilter-expr-result' )
|
|
.text( data.abusefilterevalexpression.result );
|
|
} );
|
|
}
|
|
|
|
/**
|
|
* Processes the result of the unblocking autopromotions for a user
|
|
*
|
|
* @param {Object} data The response of the API request
|
|
*/
|
|
function processReautoconfirm( data ) {
|
|
mw.notify(
|
|
mw.message(
|
|
'abusefilter-reautoconfirm-done',
|
|
data.abusefilterunblockautopromote.user
|
|
).toString()
|
|
);
|
|
|
|
$.removeSpinner( 'abusefilter-reautoconfirm' );
|
|
}
|
|
|
|
/**
|
|
* Processes the result of the unblocking autopromotions for a user in case of an error
|
|
*
|
|
* @param {string} errorCode Identifier of the error
|
|
* @param {Object} data The response of the API request
|
|
*/
|
|
function processReautoconfirmFailure( errorCode, data ) {
|
|
var msg;
|
|
|
|
switch ( errorCode ) {
|
|
case 'permissiondenied':
|
|
msg = mw.msg( 'abusefilter-reautoconfirm-notallowed' );
|
|
break;
|
|
case 'http':
|
|
msg = mw.msg( 'abusefilter-http-error', data && data.exception );
|
|
break;
|
|
case 'notsuspended':
|
|
msg = data.error.info;
|
|
break;
|
|
default:
|
|
msg = mw.msg( 'unknown-error' );
|
|
break;
|
|
}
|
|
mw.notify( msg );
|
|
|
|
$.removeSpinner( 'abusefilter-reautoconfirm' );
|
|
}
|
|
|
|
/**
|
|
* Submits a call to reautoconfirm a user.
|
|
* @context HTMLElement
|
|
* @param {jQuery.Event} e The event fired when the function is called
|
|
* @return {boolean} False to prevent form submission
|
|
*/
|
|
function doReautoSubmit() {
|
|
var nameField = OO.ui.infuse( $( '#reautoconfirm-user' ) ),
|
|
name = nameField.getValue(),
|
|
api;
|
|
|
|
if ( name === '' ) {
|
|
return false;
|
|
}
|
|
|
|
$( this ).injectSpinner( { id: 'abusefilter-reautoconfirm', size: 'large' } );
|
|
|
|
api = new mw.Api();
|
|
api.post( {
|
|
action: 'abusefilterunblockautopromote',
|
|
user: name,
|
|
token: mw.user.tokens.get( 'editToken' )
|
|
} )
|
|
.done( processReautoconfirm )
|
|
.fail( processReautoconfirmFailure );
|
|
return false;
|
|
}
|
|
|
|
$( function initialize() {
|
|
$( '#mw-abusefilter-submitexpr' ).on( 'click', doExprSubmit );
|
|
$( '#mw-abusefilter-reautoconfirmsubmit' ).on( 'click', doReautoSubmit );
|
|
} );
|
|
}() );
|