mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 18:19:38 +00:00
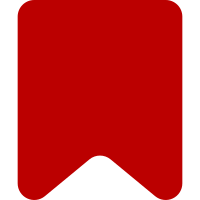
These methods had no reals reason to be static and belong to the AbuseFilter class. Most of them were moved to Parser class as common variations of the existing entry points. One was specific to the EvalExpression API module and was moved there. This change comes at no cost, and will make it possible to inject a parser where needed. Change-Id: Ifd169cfc99df8a5eb4ca94ac330f301ca28a2442
79 lines
2 KiB
PHP
79 lines
2 KiB
PHP
<?php
|
|
|
|
use MediaWiki\Extension\AbuseFilter\VariableGenerator\VariableGenerator;
|
|
|
|
class ApiAbuseFilterEvalExpression extends ApiBase {
|
|
/**
|
|
* @see ApiBase::execute()
|
|
*/
|
|
public function execute() {
|
|
// "Anti-DoS"
|
|
if ( !AbuseFilter::canViewPrivate( $this->getUser() ) ) {
|
|
$this->dieWithError( 'apierror-abusefilter-canteval', 'permissiondenied' );
|
|
}
|
|
|
|
$params = $this->extractRequestParams();
|
|
|
|
$status = $this->evaluateExpression( $params['expression'] );
|
|
if ( !$status->isGood() ) {
|
|
$this->dieWithError( $status->getErrors()[0] );
|
|
} else {
|
|
$res = $status->getValue();
|
|
$res = $params['prettyprint'] ? AbuseFilter::formatVar( $res ) : $res;
|
|
$this->getResult()->addValue(
|
|
null,
|
|
$this->getModuleName(),
|
|
ApiResult::addMetadataToResultVars( [ 'result' => $res ] )
|
|
);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @param string $expr
|
|
* @return Status
|
|
*/
|
|
private function evaluateExpression( string $expr ) : Status {
|
|
$parser = AbuseFilter::getDefaultParser();
|
|
if ( $parser->checkSyntax( $expr ) !== true ) {
|
|
return Status::newFatal( 'abusefilter-tools-syntax-error' );
|
|
}
|
|
|
|
$vars = new AbuseFilterVariableHolder();
|
|
// Generic vars are the only ones available
|
|
$generator = new VariableGenerator( $vars );
|
|
$vars = $generator->addGenericVars()->getVariableHolder();
|
|
$vars->setVar( 'timestamp', wfTimestamp( TS_UNIX ) );
|
|
$parser->setVariables( $vars );
|
|
|
|
return Status::newGood( $parser->evaluateExpression( $expr ) );
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getAllowedParams()
|
|
* @return array
|
|
*/
|
|
public function getAllowedParams() {
|
|
return [
|
|
'expression' => [
|
|
ApiBase::PARAM_REQUIRED => true,
|
|
],
|
|
'prettyprint' => [
|
|
ApiBase::PARAM_TYPE => 'boolean'
|
|
]
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getExamplesMessages()
|
|
* @return array
|
|
*/
|
|
protected function getExamplesMessages() {
|
|
return [
|
|
'action=abusefilterevalexpression&expression=lcase("FOO")'
|
|
=> 'apihelp-abusefilterevalexpression-example-1',
|
|
'action=abusefilterevalexpression&expression=lcase("FOO")&prettyprint=1'
|
|
=> 'apihelp-abusefilterevalexpression-example-2',
|
|
];
|
|
}
|
|
}
|