mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-14 09:44:44 +00:00
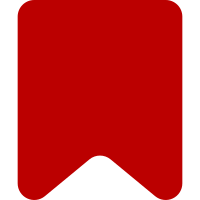
This is just a start; next step is adding a factory/store method to get/store these objects. And then use these value objects whenever applicable. Note: the actions-related code is still not fully implemented. This is going to happen as part of the FilterLookup. Change-Id: I5f33227887c035e301313bbe24d1c1fefb75bc6a
87 lines
1.4 KiB
PHP
87 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter\Filter;
|
|
|
|
/**
|
|
* (Mutable) value object to represent flags that can be *manually* set on a filter.
|
|
*/
|
|
class Flags {
|
|
/** @var bool */
|
|
private $enabled;
|
|
/** @var bool */
|
|
private $deleted;
|
|
/** @var bool */
|
|
private $hidden;
|
|
/** @var bool */
|
|
private $global;
|
|
|
|
/**
|
|
* @param bool $enabled
|
|
* @param bool $deleted
|
|
* @param bool $hidden
|
|
* @param bool $global
|
|
*/
|
|
public function __construct( bool $enabled, bool $deleted, bool $hidden, bool $global ) {
|
|
$this->enabled = $enabled;
|
|
$this->deleted = $deleted;
|
|
$this->hidden = $hidden;
|
|
$this->global = $global;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getEnabled(): bool {
|
|
return $this->enabled;
|
|
}
|
|
|
|
/**
|
|
* @param bool $enabled
|
|
*/
|
|
public function setEnabled( bool $enabled ): void {
|
|
$this->enabled = $enabled;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getDeleted(): bool {
|
|
return $this->deleted;
|
|
}
|
|
|
|
/**
|
|
* @param bool $deleted
|
|
*/
|
|
public function setDeleted( bool $deleted ): void {
|
|
$this->deleted = $deleted;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getHidden(): bool {
|
|
return $this->hidden;
|
|
}
|
|
|
|
/**
|
|
* @param bool $hidden
|
|
*/
|
|
public function setHidden( bool $hidden ): void {
|
|
$this->hidden = $hidden;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getGlobal(): bool {
|
|
return $this->global;
|
|
}
|
|
|
|
/**
|
|
* @param bool $global
|
|
*/
|
|
public function setGlobal( bool $global ): void {
|
|
$this->global = $global;
|
|
}
|
|
}
|