mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-13 17:27:20 +00:00
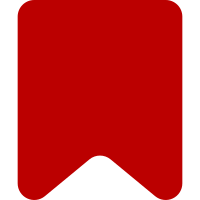
Some pieces of code were updated to use Filter objects, while other places are still to be updated. We also need to change the history part to exclude actions somehow, cleanup the ViewEdit, reduce direct DB access or anything mentioning DB fields outside of FilterLookup, etc. Change-Id: I42b7ded685db76eddd45e4b1336f9828cba811ce
68 lines
1.7 KiB
PHP
68 lines
1.7 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter;
|
|
|
|
use Wikimedia\Rdbms\DBError;
|
|
use Wikimedia\Rdbms\IDatabase;
|
|
use Wikimedia\Rdbms\LBFactory;
|
|
|
|
class CentralDBManager {
|
|
public const SERVICE_NAME = 'AbuseFilterCentralDBManager';
|
|
|
|
/** @var LBFactory */
|
|
private $loadBalancerFactory;
|
|
/** @var string|null */
|
|
private $dbName;
|
|
/** @var bool */
|
|
private $filterIsCentral;
|
|
|
|
/**
|
|
* @param LBFactory $loadBalancerFactory
|
|
* @param string|false|null $dbName
|
|
* @param bool $filterIsCentral
|
|
*/
|
|
public function __construct( LBFactory $loadBalancerFactory, $dbName, bool $filterIsCentral ) {
|
|
$this->loadBalancerFactory = $loadBalancerFactory;
|
|
// Use false to agree with LoadBalancer
|
|
$this->dbName = $dbName ?: false;
|
|
$this->filterIsCentral = $filterIsCentral;
|
|
}
|
|
|
|
/**
|
|
* @param int $index DB_MASTER/DB_REPLICA
|
|
* @return IDatabase
|
|
* @throws DBError
|
|
* @throws CentralDBNotAvailableException
|
|
*/
|
|
public function getConnection( int $index ) : IDatabase {
|
|
if ( !is_string( $this->dbName ) ) {
|
|
throw new CentralDBNotAvailableException( '$wgAbuseFilterCentralDB is not configured' );
|
|
}
|
|
|
|
return $this->loadBalancerFactory
|
|
->getMainLB( $this->dbName )
|
|
->getConnectionRef( $index, [], $this->dbName );
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
* @throws CentralDBNotAvailableException
|
|
*/
|
|
public function getCentralDBName() : string {
|
|
if ( !is_string( $this->dbName ) ) {
|
|
throw new CentralDBNotAvailableException( '$wgAbuseFilterCentralDB is not configured' );
|
|
}
|
|
return $this->dbName;
|
|
}
|
|
|
|
/**
|
|
* Whether this database is the central one.
|
|
* @todo Deprecate the config in favour of just checking whether the current DB is the same
|
|
* as $wgAbuseFilterCentralDB.
|
|
* @return bool
|
|
*/
|
|
public function filterIsCentral() : bool {
|
|
return $this->filterIsCentral;
|
|
}
|
|
}
|