mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-24 22:15:26 +00:00
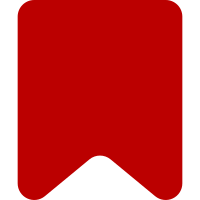
- to compensate for the slower parser, optimised it a little - change basic_string to fray, a refcounted immutable string - add 'xml' tool, prints the parser tree in XML
58 lines
1.2 KiB
C++
58 lines
1.2 KiB
C++
/*
|
|
* Copyright (c) 2008 Andrew Garrett.
|
|
* Copyright (c) 2008 River Tarnell <river@wikimedia.org>
|
|
* Derived from public domain code contributed by Victor Vasiliev.
|
|
*
|
|
* Permission is granted to anyone to use this software for any purpose,
|
|
* including commercial applications, and to alter it and redistribute it
|
|
* freely. This software is provided 'as-is', without any express or
|
|
* implied warranty.
|
|
*/
|
|
|
|
#include <cstdlib>
|
|
#include <iostream>
|
|
#include <iterator>
|
|
#include <string>
|
|
#include <sstream>
|
|
#include <fstream>
|
|
#include <map>
|
|
#include <cerrno>
|
|
#include <cstring>
|
|
|
|
#include <boost/format.hpp>
|
|
#include <boost/next_prior.hpp>
|
|
|
|
#include "request.h"
|
|
|
|
int main( int argc, char** argv ) {
|
|
afp::request r;
|
|
|
|
while (true) {
|
|
bool result = false;
|
|
|
|
try {
|
|
if (argv[1]) {
|
|
std::ifstream inf(argv[1]);
|
|
if (!inf) {
|
|
std::cerr << boost::format("%s: %s: %s\n")
|
|
% argv[0] % argv[1] % std::strerror(errno);
|
|
return 0;
|
|
}
|
|
|
|
if (!r.load(inf))
|
|
return 0;
|
|
} else {
|
|
if (!r.load(std::cin))
|
|
return 0;
|
|
}
|
|
|
|
result = r.evaluate();
|
|
} catch (afp::exception &excep) {
|
|
std::cerr << "EXCEPTION: " << excep.what() << std::endl;
|
|
}
|
|
|
|
std::cout << ( result ? "MATCH\n" : "NOMATCH\n" );
|
|
}
|
|
}
|
|
|