mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-13 17:27:20 +00:00
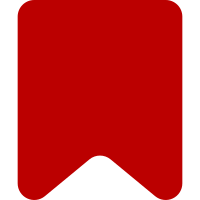
List which actions were disabled, or explicitly say that no actions were disabled if that's the case. Also avoid the word "throttle" in messages as it may be hard to translate. Also don't suggest optimizations to the filter conditions -- unoptimized rules have nothing to do with a filter being throttled. Bug: T200036 Change-Id: Id989fb185453d068b7685241ee49189a2df67b5f
66 lines
1.8 KiB
PHP
66 lines
1.8 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter;
|
|
|
|
use EchoEventPresentationModel;
|
|
use Message;
|
|
|
|
class ThrottleFilterPresentationModel extends EchoEventPresentationModel {
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getIconType() {
|
|
return 'placeholder';
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getHeaderMessage() {
|
|
$text = $this->event->getTitle()->getText();
|
|
list( , $filter ) = explode( '/', $text, 2 );
|
|
$disabledActions = $this->event->getExtraParam( 'throttled-actions' );
|
|
if ( $disabledActions === null ) {
|
|
// BC for when we didn't include the actions here.
|
|
return $this->msg( 'notification-header-throttle-filter' )
|
|
->params( $this->getViewingUserForGender() )
|
|
->numParams( $filter );
|
|
}
|
|
if ( $disabledActions ) {
|
|
$specsFormatter = AbuseFilterServices::getSpecsFormatter();
|
|
$specsFormatter->setMessageLocalizer( $this );
|
|
$disabledActionsLocalized = [];
|
|
foreach ( $disabledActions as $action ) {
|
|
$disabledActionsLocalized[] = $specsFormatter->getActionMessage( $action )->text();
|
|
}
|
|
return $this->msg( 'notification-header-throttle-filter-actions' )
|
|
->params( $this->getViewingUserForGender() )
|
|
->numParams( $filter )
|
|
->params( Message::listParam( $disabledActionsLocalized ) )
|
|
->params( count( $disabledActionsLocalized ) );
|
|
}
|
|
return $this->msg( 'notification-header-throttle-filter-no-actions' )
|
|
->params( $this->getViewingUserForGender() )
|
|
->numParams( $filter );
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getSubjectMessage() {
|
|
return $this->msg( 'notification-subject-throttle-filter' )
|
|
->params( $this->getViewingUserForGender() );
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getPrimaryLink() {
|
|
return [
|
|
'url' => $this->event->getTitle()->getFullURL(),
|
|
'label' => $this->msg( 'notification-link-text-show-filter' )->text()
|
|
];
|
|
}
|
|
}
|