mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-24 22:15:26 +00:00
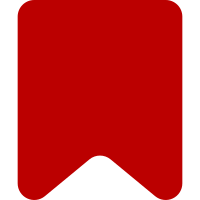
af_actions and af_hidden are treated in the same way, so avoid duplicating that code. Some of the remaining cases are also quite similar (although not identical), so we might want to merge them in the future. Change-Id: I1b48502e077e58eb9ff459326bba18bb1d127242
72 lines
2 KiB
PHP
72 lines
2 KiB
PHP
<?php
|
|
|
|
use MediaWiki\Linker\LinkRenderer;
|
|
|
|
/**
|
|
* Class to build paginated filter list for wikis using global abuse filters
|
|
*/
|
|
class GlobalAbuseFilterPager extends AbuseFilterPager {
|
|
/**
|
|
* @param AbuseFilterViewList $page
|
|
* @param array $conds
|
|
* @param LinkRenderer $linkRenderer
|
|
*/
|
|
public function __construct( AbuseFilterViewList $page, $conds, LinkRenderer $linkRenderer ) {
|
|
parent::__construct( $page, $conds, $linkRenderer, null, null );
|
|
$this->mDb = wfGetDB(
|
|
DB_REPLICA, [], $this->getConfig()->get( 'AbuseFilterCentralDB' ) );
|
|
}
|
|
|
|
/**
|
|
* @param string $name
|
|
* @param string $value
|
|
* @return string
|
|
*/
|
|
public function formatValue( $name, $value ) {
|
|
$lang = $this->getLanguage();
|
|
$row = $this->mCurrentRow;
|
|
|
|
switch ( $name ) {
|
|
case 'af_id':
|
|
return $lang->formatNum( intval( $value ) );
|
|
case 'af_public_comments':
|
|
return $this->getOutput()->parseInlineAsInterface( $value );
|
|
case 'af_enabled':
|
|
$statuses = [];
|
|
if ( $row->af_deleted ) {
|
|
$statuses[] = $this->msg( 'abusefilter-deleted' )->parse();
|
|
} elseif ( $row->af_enabled ) {
|
|
$statuses[] = $this->msg( 'abusefilter-enabled' )->parse();
|
|
} else {
|
|
$statuses[] = $this->msg( 'abusefilter-disabled' )->parse();
|
|
}
|
|
if ( $row->af_global ) {
|
|
$statuses[] = $this->msg( 'abusefilter-status-global' )->parse();
|
|
}
|
|
|
|
return $lang->commaList( $statuses );
|
|
case 'af_hit_count':
|
|
// If the rule is hidden, don't show it, even to priviledged local admins
|
|
if ( $row->af_hidden ) {
|
|
return '';
|
|
}
|
|
return $this->msg( 'abusefilter-hitcount' )->numParams( $value )->parse();
|
|
case 'af_timestamp':
|
|
$user = $row->af_user_text;
|
|
return $this->msg(
|
|
'abusefilter-edit-lastmod-text',
|
|
$lang->timeanddate( $value, true ),
|
|
$user,
|
|
$lang->date( $value, true ),
|
|
$lang->time( $value, true ),
|
|
$user
|
|
)->parse();
|
|
case 'af_group':
|
|
// If this is global, local name probably doesn't exist, but try
|
|
return AbuseFilter::nameGroup( $value );
|
|
default:
|
|
return parent::formatValue( $name, $value );
|
|
}
|
|
}
|
|
}
|