mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 18:19:38 +00:00
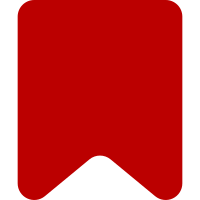
Some pieces of code were updated to use Filter objects, while other places are still to be updated. We also need to change the history part to exclude actions somehow, cleanup the ViewEdit, reduce direct DB access or anything mentioning DB fields outside of FilterLookup, etc. Change-Id: I42b7ded685db76eddd45e4b1336f9828cba811ce
1361 lines
42 KiB
PHP
1361 lines
42 KiB
PHP
<?php
|
|
|
|
use MediaWiki\Extension\AbuseFilter\AbuseFilterServices;
|
|
use MediaWiki\Extension\AbuseFilter\Filter\Filter;
|
|
use MediaWiki\Extension\AbuseFilter\Filter\FilterNotFoundException;
|
|
use MediaWiki\Extension\AbuseFilter\Filter\FilterVersionNotFoundException;
|
|
use MediaWiki\Extension\AbuseFilter\Filter\Flags;
|
|
use MediaWiki\Extension\AbuseFilter\Filter\LastEditInfo;
|
|
use MediaWiki\Extension\AbuseFilter\Filter\MutableFilter;
|
|
use MediaWiki\Extension\AbuseFilter\Filter\Specs;
|
|
use MediaWiki\Extension\AbuseFilter\FilterLookup;
|
|
use MediaWiki\Linker\LinkRenderer;
|
|
use MediaWiki\MediaWikiServices;
|
|
|
|
class AbuseFilterViewEdit extends AbuseFilterView {
|
|
// Temporary hack
|
|
private const EXPORT_EXCLUDED_PROPS = [
|
|
'af_id', 'af_timestamp', 'af_user', 'af_user_text', 'af_hit_count', 'af_throttled'
|
|
];
|
|
/**
|
|
* @var int|null The history ID of the current filter
|
|
*/
|
|
private $historyID;
|
|
/** @var int|string */
|
|
private $filter;
|
|
|
|
/**
|
|
* @param IContextSource $context
|
|
* @param LinkRenderer $linkRenderer
|
|
* @param string $basePageName
|
|
* @param array $params
|
|
*/
|
|
public function __construct(
|
|
IContextSource $context,
|
|
LinkRenderer $linkRenderer,
|
|
string $basePageName,
|
|
array $params
|
|
) {
|
|
parent::__construct( $context, $linkRenderer, $basePageName, $params );
|
|
$this->filter = $this->mParams['filter'];
|
|
$this->historyID = $this->mParams['history'] ?? null;
|
|
}
|
|
|
|
/**
|
|
* Shows the page
|
|
*/
|
|
public function show() {
|
|
$user = $this->getUser();
|
|
$out = $this->getOutput();
|
|
$out->enableOOUI();
|
|
$request = $this->getRequest();
|
|
$afPermManager = AbuseFilterServices::getPermissionManager();
|
|
$out->setPageTitle( $this->msg( 'abusefilter-edit' ) );
|
|
$out->addHelpLink( 'Extension:AbuseFilter/Rules format' );
|
|
|
|
$filter = $this->filter;
|
|
if ( !is_numeric( $filter ) && $filter !== null ) {
|
|
$this->showUnrecoverableError( 'abusefilter-edit-badfilter' );
|
|
return;
|
|
}
|
|
$history_id = $this->historyID;
|
|
if ( $this->historyID ) {
|
|
$dbr = wfGetDB( DB_REPLICA );
|
|
$lastID = (int)$dbr->selectField(
|
|
'abuse_filter_history',
|
|
'afh_id',
|
|
[
|
|
'afh_filter' => $filter,
|
|
],
|
|
__METHOD__,
|
|
[ 'ORDER BY' => 'afh_id DESC' ]
|
|
);
|
|
// change $history_id to null if it's current version id
|
|
if ( $lastID === $this->historyID ) {
|
|
$history_id = null;
|
|
}
|
|
}
|
|
|
|
// Add the default warning and disallow messages in a JS variable
|
|
$this->exposeMessages();
|
|
|
|
$canEdit = $afPermManager->canEdit( $user );
|
|
|
|
if ( $filter === null && !$canEdit ) {
|
|
// Special case: Special:AbuseFilter/new is certainly not usable if the user cannot edit
|
|
$this->showUnrecoverableError( 'abusefilter-edit-notallowed' );
|
|
return;
|
|
}
|
|
|
|
$isImport = $request->wasPosted() && $request->getRawVal( 'wpImportText' ) !== null;
|
|
|
|
if ( !$isImport && $request->wasPosted() && $canEdit ) {
|
|
$this->attemptSave( $filter, $history_id );
|
|
return;
|
|
}
|
|
|
|
if ( $isImport ) {
|
|
$filterObj = $this->loadImportRequest();
|
|
if ( $filterObj === null ) {
|
|
$this->showUnrecoverableError( 'abusefilter-import-invalid-data' );
|
|
return;
|
|
}
|
|
} else {
|
|
// The request wasn't posted (i.e. just viewing the filter) or the user cannot edit
|
|
try {
|
|
$filterObj = $this->loadFromDatabase( $filter, $history_id );
|
|
} catch ( FilterNotFoundException $_ ) {
|
|
$filterObj = null;
|
|
}
|
|
if ( $filterObj === null || ( $history_id && (int)$filterObj->getID() !== $filter ) ) {
|
|
$this->showUnrecoverableError( 'abusefilter-edit-badfilter' );
|
|
return;
|
|
}
|
|
}
|
|
|
|
$this->buildFilterEditor( null, $filterObj, $filter, $history_id );
|
|
}
|
|
|
|
/**
|
|
* @param int|null $filter The filter ID or null for a new filter
|
|
* @param int|null $history_id The history ID of the filter, if applicable. Otherwise null
|
|
*/
|
|
private function attemptSave( ?int $filter, $history_id ) : void {
|
|
$out = $this->getOutput();
|
|
$request = $this->getRequest();
|
|
$user = $this->getUser();
|
|
|
|
[ $newFilter, $origFilter ] = $this->loadRequest( $filter );
|
|
|
|
$tokenFilter = $filter === null ? 'new' : (string)$filter;
|
|
$editToken = $request->getVal( 'wpEditToken' );
|
|
$tokenMatches = $user->matchEditToken(
|
|
$editToken, [ 'abusefilter', $tokenFilter ], $request );
|
|
|
|
if ( !$tokenMatches ) {
|
|
// Token invalid or expired while the page was open, warn to retry
|
|
$error = Html::warningBox( $this->msg( 'abusefilter-edit-token-not-match' )->parseAsBlock() );
|
|
$this->buildFilterEditor( $error, $newFilter, $filter, $history_id );
|
|
return;
|
|
}
|
|
|
|
$dbw = wfGetDB( DB_MASTER );
|
|
$status = AbuseFilter::saveFilter(
|
|
$user, $filter, $newFilter,
|
|
$origFilter, $dbw, $this->getConfig()
|
|
);
|
|
|
|
if ( !$status->isGood() ) {
|
|
$errors = $status->getErrors();
|
|
[ 'message' => $msg, 'params' => $params ] = $errors[0];
|
|
if ( $status->isOK() ) {
|
|
// Fixable error, show the editing interface
|
|
$error = Html::errorBox( $this->msg( $msg, $params )->parseAsBlock() );
|
|
$this->buildFilterEditor( $error, $newFilter, $filter, $history_id );
|
|
} else {
|
|
$this->showUnrecoverableError( $msg );
|
|
}
|
|
} elseif ( $status->getValue() === false ) {
|
|
// No change
|
|
$out->redirect( $this->getTitle()->getLocalURL() );
|
|
} else {
|
|
// Everything went fine!
|
|
list( $new_id, $history_id ) = $status->getValue();
|
|
$out->redirect(
|
|
$this->getTitle()->getLocalURL(
|
|
[
|
|
'result' => 'success',
|
|
'changedfilter' => $new_id,
|
|
'changeid' => $history_id,
|
|
]
|
|
)
|
|
);
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @param string $msgKey
|
|
*/
|
|
private function showUnrecoverableError( string $msgKey ) : void {
|
|
$out = $this->getOutput();
|
|
|
|
$out->addHTML( Html::errorBox( $this->msg( $msgKey )->parseAsBlock() ) );
|
|
$href = $this->getTitle()->getFullURL();
|
|
$btn = new OOUI\ButtonWidget( [
|
|
'label' => $this->msg( 'abusefilter-return' )->text(),
|
|
'href' => $href
|
|
] );
|
|
$out->addHTML( $btn );
|
|
}
|
|
|
|
/**
|
|
* Builds the full form for edit filters, adding it to the OutputPage. This method can be called in 5 different
|
|
* situations, for a total of 5 different data sources for $filterObj and $actions:
|
|
* 1 - View the result of importing a filter
|
|
* 2 - Create a new filter
|
|
* 3 - Load the current version of an existing filter
|
|
* 4 - Load an old version of an existing filter
|
|
* 5 - Show the user input again if saving fails after one of the steps above
|
|
*
|
|
* @param string|null $error An error message to show above the filter box (HTML).
|
|
* @param Filter $filterObj
|
|
* @param int|null $filter The filter ID, or null for a new filter
|
|
* @param int|null $history_id The history ID of the filter, if applicable. Otherwise null
|
|
*/
|
|
protected function buildFilterEditor(
|
|
$error,
|
|
Filter $filterObj,
|
|
?int $filter,
|
|
$history_id
|
|
) {
|
|
$out = $this->getOutput();
|
|
$out->addJsConfigVars( 'isFilterEditor', true );
|
|
$lang = $this->getLanguage();
|
|
$user = $this->getUser();
|
|
$afPermManager = AbuseFilterServices::getPermissionManager();
|
|
$actions = $filterObj->getActions();
|
|
|
|
$out->addSubtitle( $this->msg(
|
|
$filter === null ? 'abusefilter-edit-subtitle-new' : 'abusefilter-edit-subtitle',
|
|
$filter === null ? $filter : $this->getLanguage()->formatNum( $filter ),
|
|
$history_id
|
|
)->parse() );
|
|
|
|
// We use filterHidden() to ensure that if a public filter is made private, the public
|
|
// revision is also hidden.
|
|
if (
|
|
( $filterObj->isHidden() || ( $filter !== null && AbuseFilter::filterHidden( $filter ) ) ) &&
|
|
!$afPermManager->canViewPrivateFilters( $user )
|
|
) {
|
|
$out->addHTML( $this->msg( 'abusefilter-edit-denied' )->escaped() );
|
|
return;
|
|
}
|
|
|
|
$readOnly = !$afPermManager->canEditFilter( $user, $filterObj );
|
|
|
|
if ( $history_id ) {
|
|
$oldWarningMessage = $readOnly
|
|
? 'abusefilter-edit-oldwarning-view'
|
|
: 'abusefilter-edit-oldwarning';
|
|
$out->addWikiMsg( $oldWarningMessage, $history_id, $filter );
|
|
}
|
|
|
|
if ( $error !== null ) {
|
|
$out->addHTML( $error );
|
|
}
|
|
|
|
$fields = [];
|
|
|
|
$fields['abusefilter-edit-id'] =
|
|
$filter === null ?
|
|
$this->msg( 'abusefilter-edit-new' )->escaped() :
|
|
$lang->formatNum( (string)$filter );
|
|
$fields['abusefilter-edit-description'] =
|
|
new OOUI\TextInputWidget( [
|
|
'name' => 'wpFilterDescription',
|
|
'value' => $filterObj->getName(),
|
|
'readOnly' => $readOnly
|
|
]
|
|
);
|
|
|
|
$validGroups = $this->getConfig()->get( 'AbuseFilterValidGroups' );
|
|
if ( count( $validGroups ) > 1 ) {
|
|
$groupSelector =
|
|
new OOUI\DropdownInputWidget( [
|
|
'name' => 'wpFilterGroup',
|
|
'id' => 'mw-abusefilter-edit-group-input',
|
|
'value' => $filterObj->getGroup(),
|
|
'disabled' => $readOnly
|
|
] );
|
|
|
|
$options = [];
|
|
foreach ( $validGroups as $group ) {
|
|
$options += [ AbuseFilter::nameGroup( $group ) => $group ];
|
|
}
|
|
|
|
$options = Xml::listDropDownOptionsOoui( $options );
|
|
$groupSelector->setOptions( $options );
|
|
|
|
$fields['abusefilter-edit-group'] = $groupSelector;
|
|
}
|
|
|
|
// Hit count display
|
|
if ( $filterObj->getHitCount() !== null && $afPermManager->canSeeLogDetails( $user ) ) {
|
|
$count_display = $this->msg( 'abusefilter-hitcount' )
|
|
->numParams( $filterObj->getHitCount() )->text();
|
|
$hitCount = $this->linkRenderer->makeKnownLink(
|
|
SpecialPage::getTitleFor( 'AbuseLog' ),
|
|
$count_display,
|
|
[],
|
|
[ 'wpSearchFilter' => $filterObj->getID() ]
|
|
);
|
|
|
|
$fields['abusefilter-edit-hitcount'] = $hitCount;
|
|
}
|
|
|
|
if ( $filter !== null && $filterObj->isEnabled() ) {
|
|
// Statistics
|
|
[
|
|
'count' => $totalCount,
|
|
'matches' => $matchesCount,
|
|
'total-time' => $curTotalTime,
|
|
'total-cond' => $curTotalConds,
|
|
] = AbuseFilterServices::getFilterProfiler()->getFilterProfile( $filter );
|
|
|
|
if ( $totalCount > 0 ) {
|
|
$matchesPercent = round( 100 * $matchesCount / $totalCount, 2 );
|
|
$avgTime = round( $curTotalTime / $totalCount, 2 );
|
|
$avgCond = round( $curTotalConds / $totalCount, 1 );
|
|
|
|
$fields['abusefilter-edit-status-label'] = $this->msg( 'abusefilter-edit-status' )
|
|
->numParams( $totalCount, $matchesCount, $matchesPercent, $avgTime, $avgCond )
|
|
->parse();
|
|
}
|
|
}
|
|
|
|
$fields['abusefilter-edit-rules'] = $this->buildEditBox(
|
|
$filterObj->getRules(),
|
|
true
|
|
);
|
|
$fields['abusefilter-edit-notes'] =
|
|
new OOUI\MultilineTextInputWidget( [
|
|
'name' => 'wpFilterNotes',
|
|
'value' => $filterObj->getComments() . "\n",
|
|
'rows' => 15,
|
|
'readOnly' => $readOnly,
|
|
'id' => 'mw-abusefilter-notes-editor'
|
|
] );
|
|
|
|
// Build checkboxes
|
|
$checkboxes = [ 'hidden', 'enabled', 'deleted' ];
|
|
$flags = '';
|
|
|
|
if ( $this->getConfig()->get( 'AbuseFilterIsCentral' ) ) {
|
|
$checkboxes[] = 'global';
|
|
}
|
|
|
|
if ( $filterObj->isThrottled() ) {
|
|
$throttledActions = array_intersect(
|
|
$filterObj->getActionsNames(),
|
|
AbuseFilter::getDangerousActions()
|
|
);
|
|
|
|
if ( $throttledActions ) {
|
|
$throttledActions = array_map(
|
|
function ( $filterAction ) {
|
|
// TODO: This is AbuseFilter::getActionDisplay, but not escaped
|
|
return $this->msg( 'abusefilter-action-' . $filterAction )->text();
|
|
},
|
|
$throttledActions
|
|
);
|
|
|
|
$flags .= Html::warningBox(
|
|
$this->msg( 'abusefilter-edit-throttled-warning' )
|
|
->plaintextParams( $lang->commaList( $throttledActions ) )
|
|
->parseAsBlock()
|
|
);
|
|
}
|
|
}
|
|
|
|
foreach ( $checkboxes as $checkboxId ) {
|
|
// Messages that can be used here:
|
|
// * abusefilter-edit-enabled
|
|
// * abusefilter-edit-deleted
|
|
// * abusefilter-edit-hidden
|
|
// * abusefilter-edit-global
|
|
$message = "abusefilter-edit-$checkboxId";
|
|
// isEnabled(), isDeleted(), isHidden(), isGlobal()
|
|
$method = 'is' . ucfirst( $checkboxId );
|
|
$postVar = 'wpFilter' . ucfirst( $checkboxId );
|
|
|
|
$checkboxAttribs = [
|
|
'name' => $postVar,
|
|
'id' => $postVar,
|
|
'selected' => $filterObj->$method(),
|
|
'disabled' => $readOnly
|
|
];
|
|
$labelAttribs = [
|
|
'label' => $this->msg( $message )->text(),
|
|
'align' => 'inline',
|
|
];
|
|
|
|
if ( $checkboxId === 'global' && !$afPermManager->canEditGlobal( $user ) ) {
|
|
$checkboxAttribs['disabled'] = 'disabled';
|
|
}
|
|
|
|
// Set readonly on deleted if the filter isn't disabled
|
|
if ( $checkboxId === 'deleted' && $filterObj->isEnabled() ) {
|
|
$checkboxAttribs['disabled'] = 'disabled';
|
|
}
|
|
|
|
// Add infusable where needed
|
|
if ( $checkboxId === 'deleted' || $checkboxId === 'enabled' ) {
|
|
$checkboxAttribs['infusable'] = true;
|
|
if ( $checkboxId === 'deleted' ) {
|
|
$labelAttribs['id'] = $postVar . 'Label';
|
|
$labelAttribs['infusable'] = true;
|
|
}
|
|
}
|
|
|
|
$checkbox =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\CheckboxInputWidget( $checkboxAttribs ),
|
|
$labelAttribs
|
|
);
|
|
$flags .= $checkbox;
|
|
}
|
|
|
|
$fields['abusefilter-edit-flags'] = $flags;
|
|
|
|
if ( $filter !== null ) {
|
|
$tools = '';
|
|
if ( $afPermManager->canRevertFilterActions( $user ) ) {
|
|
$tools .= Xml::tags(
|
|
'p', null,
|
|
$this->linkRenderer->makeLink(
|
|
$this->getTitle( "revert/$filter" ),
|
|
new HtmlArmor( $this->msg( 'abusefilter-edit-revert' )->parse() )
|
|
)
|
|
);
|
|
}
|
|
|
|
if ( $afPermManager->canViewPrivateFilters( $user ) ) {
|
|
// Test link
|
|
$tools .= Xml::tags(
|
|
'p', null,
|
|
$this->linkRenderer->makeLink(
|
|
$this->getTitle( "test/$filter" ),
|
|
new HtmlArmor( $this->msg( 'abusefilter-edit-test-link' )->parse() )
|
|
)
|
|
);
|
|
}
|
|
// Last modification details
|
|
$userLink =
|
|
Linker::userLink( $filterObj->getUserID(), $filterObj->getUserName() ) .
|
|
Linker::userToolLinks( $filterObj->getUserID(), $filterObj->getUserName() );
|
|
$fields['abusefilter-edit-lastmod'] =
|
|
$this->msg( 'abusefilter-edit-lastmod-text' )
|
|
->rawParams(
|
|
$this->getLinkToLatestDiff(
|
|
$filter,
|
|
$lang->timeanddate( $filterObj->getTimestamp(), true )
|
|
),
|
|
$userLink,
|
|
$this->getLinkToLatestDiff(
|
|
$filter,
|
|
$lang->date( $filterObj->getTimestamp(), true )
|
|
),
|
|
$this->getLinkToLatestDiff(
|
|
$filter,
|
|
$lang->time( $filterObj->getTimestamp(), true )
|
|
)
|
|
)->params(
|
|
wfEscapeWikiText( $filterObj->getUserName() )
|
|
)->parse();
|
|
$history_display = new HtmlArmor( $this->msg( 'abusefilter-edit-viewhistory' )->parse() );
|
|
$fields['abusefilter-edit-history'] =
|
|
$this->linkRenderer->makeKnownLink( $this->getTitle( 'history/' . $filter ), $history_display );
|
|
|
|
// Add export
|
|
$exportRow = (object)array_diff_key(
|
|
get_object_vars( $filterObj->toDatabaseRow() ),
|
|
array_fill_keys( self::EXPORT_EXCLUDED_PROPS, 1 )
|
|
);
|
|
$exportText = FormatJson::encode( [ 'row' => $exportRow, 'actions' => $actions ] );
|
|
$tools .= Xml::tags( 'a', [ 'href' => '#', 'id' => 'mw-abusefilter-export-link' ],
|
|
$this->msg( 'abusefilter-edit-export' )->parse() );
|
|
$tools .=
|
|
new OOUI\MultilineTextInputWidget( [
|
|
'id' => 'mw-abusefilter-export',
|
|
'readOnly' => true,
|
|
'value' => $exportText,
|
|
'rows' => 10
|
|
] );
|
|
|
|
$fields['abusefilter-edit-tools'] = $tools;
|
|
}
|
|
|
|
$form = Xml::buildForm( $fields );
|
|
$form = Xml::fieldset( $this->msg( 'abusefilter-edit-main' )->text(), $form );
|
|
$form .= Xml::fieldset(
|
|
$this->msg( 'abusefilter-edit-consequences' )->text(),
|
|
$this->buildConsequenceEditor( $filterObj, $actions )
|
|
);
|
|
|
|
$urlFilter = $filter === null ? 'new' : (string)$filter;
|
|
if ( !$readOnly ) {
|
|
$form .=
|
|
new OOUI\ButtonInputWidget( [
|
|
'type' => 'submit',
|
|
'label' => $this->msg( 'abusefilter-edit-save' )->text(),
|
|
'useInputTag' => true,
|
|
'accesskey' => 's',
|
|
'flags' => [ 'progressive', 'primary' ]
|
|
] );
|
|
$form .= Html::hidden(
|
|
'wpEditToken',
|
|
$user->getEditToken( [ 'abusefilter', $urlFilter ] )
|
|
);
|
|
}
|
|
|
|
$form = Xml::tags( 'form',
|
|
[
|
|
'action' => $this->getTitle( $urlFilter )->getFullURL(),
|
|
'method' => 'post',
|
|
'id' => 'mw-abusefilter-editing-form'
|
|
],
|
|
$form
|
|
);
|
|
|
|
$out->addHTML( $form );
|
|
|
|
if ( $history_id ) {
|
|
// @phan-suppress-next-line PhanPossiblyUndeclaredVariable
|
|
$out->addWikiMsg( $oldWarningMessage, $history_id, $filter );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Builds the "actions" editor for a given filter.
|
|
* @param Filter $filterObj
|
|
* @param array[] $actions Array of rows from the abuse_filter_action table
|
|
* corresponding to the filter object
|
|
* @return string HTML text for an action editor.
|
|
*/
|
|
private function buildConsequenceEditor( Filter $filterObj, array $actions ) {
|
|
$enabledActions = array_filter(
|
|
$this->getConfig()->get( 'AbuseFilterActions' )
|
|
);
|
|
|
|
$setActions = [];
|
|
foreach ( $enabledActions as $action => $_ ) {
|
|
$setActions[$action] = array_key_exists( $action, $actions );
|
|
}
|
|
|
|
$output = '';
|
|
|
|
foreach ( $enabledActions as $action => $_ ) {
|
|
$params = $actions[$action] ?? null;
|
|
$output .= $this->buildConsequenceSelector(
|
|
$action, $setActions[$action], $filterObj, $params );
|
|
}
|
|
|
|
return $output;
|
|
}
|
|
|
|
/**
|
|
* @param string $action The action to build an editor for
|
|
* @param bool $set Whether or not the action is activated
|
|
* @param Filter $filterObj
|
|
* @param string[]|null $parameters Action parameters. Null iff $set is false.
|
|
* @return string|\OOUI\FieldLayout
|
|
*/
|
|
private function buildConsequenceSelector( $action, $set, $filterObj, ?array $parameters ) {
|
|
$config = $this->getConfig();
|
|
$user = $this->getUser();
|
|
$afPermManager = AbuseFilterServices::getPermissionManager();
|
|
$actions = $config->get( 'AbuseFilterActions' );
|
|
if ( empty( $actions[$action] ) ) {
|
|
return '';
|
|
}
|
|
|
|
$readOnly = !$afPermManager->canEditFilter( $user, $filterObj );
|
|
|
|
switch ( $action ) {
|
|
case 'throttle':
|
|
// Throttling is only available via object caching
|
|
if ( $config->get( 'MainCacheType' ) === CACHE_NONE ) {
|
|
return '';
|
|
}
|
|
$throttleSettings =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\CheckboxInputWidget( [
|
|
'name' => 'wpFilterActionThrottle',
|
|
'id' => 'mw-abusefilter-action-checkbox-throttle',
|
|
'selected' => $set,
|
|
'classes' => [ 'mw-abusefilter-action-checkbox' ],
|
|
'disabled' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-action-throttle' )->text(),
|
|
'align' => 'inline'
|
|
]
|
|
);
|
|
$throttleFields = [];
|
|
|
|
if ( $set ) {
|
|
// @phan-suppress-next-line PhanTypeArraySuspiciousNullable $parameters is array here
|
|
list( $throttleCount, $throttlePeriod ) = explode( ',', $parameters[1], 2 );
|
|
|
|
$throttleGroups = array_slice( $parameters, 2 );
|
|
} else {
|
|
$throttleCount = 3;
|
|
$throttlePeriod = 60;
|
|
|
|
$throttleGroups = [ 'user' ];
|
|
}
|
|
|
|
$throttleFields['abusefilter-edit-throttle-count'] =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\TextInputWidget( [
|
|
'type' => 'number',
|
|
'name' => 'wpFilterThrottleCount',
|
|
'value' => $throttleCount,
|
|
'readOnly' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-throttle-count' )->text()
|
|
]
|
|
);
|
|
$throttleFields['abusefilter-edit-throttle-period'] =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\TextInputWidget( [
|
|
'type' => 'number',
|
|
'name' => 'wpFilterThrottlePeriod',
|
|
'value' => $throttlePeriod,
|
|
'readOnly' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-throttle-period' )->text()
|
|
]
|
|
);
|
|
|
|
$groupsHelpLink = Html::element(
|
|
'a',
|
|
[
|
|
'href' => 'https://www.mediawiki.org/wiki/Special:MyLanguage/' .
|
|
'Extension:AbuseFilter/Actions#Throttling',
|
|
'target' => '_blank'
|
|
],
|
|
$this->msg( 'abusefilter-edit-throttle-groups-help-text' )->text()
|
|
);
|
|
$groupsHelp = $this->msg( 'abusefilter-edit-throttle-groups-help' )
|
|
->rawParams( $groupsHelpLink )->escaped();
|
|
$hiddenGroups =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\MultilineTextInputWidget( [
|
|
'name' => 'wpFilterThrottleGroups',
|
|
'value' => implode( "\n", $throttleGroups ),
|
|
'rows' => 5,
|
|
'placeholder' => $this->msg( 'abusefilter-edit-throttle-hidden-placeholder' )->text(),
|
|
'infusable' => true,
|
|
'id' => 'mw-abusefilter-hidden-throttle-field',
|
|
'readOnly' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => new OOUI\HtmlSnippet(
|
|
$this->msg( 'abusefilter-edit-throttle-groups' )->parse()
|
|
),
|
|
'align' => 'top',
|
|
'id' => 'mw-abusefilter-hidden-throttle',
|
|
'help' => new OOUI\HtmlSnippet( $groupsHelp ),
|
|
'helpInline' => true
|
|
]
|
|
);
|
|
|
|
$throttleFields['abusefilter-edit-throttle-groups'] = $hiddenGroups;
|
|
|
|
$throttleConfig = [
|
|
'values' => $throttleGroups,
|
|
'label' => $this->msg( 'abusefilter-edit-throttle-groups' )->parse(),
|
|
'disabled' => $readOnly,
|
|
'help' => $groupsHelp
|
|
];
|
|
$this->getOutput()->addJsConfigVars( 'throttleConfig', $throttleConfig );
|
|
|
|
$throttleSettings .=
|
|
Xml::tags(
|
|
'div',
|
|
[ 'id' => 'mw-abusefilter-throttle-parameters' ],
|
|
new OOUI\FieldsetLayout( [ 'items' => $throttleFields ] )
|
|
);
|
|
return $throttleSettings;
|
|
case 'disallow':
|
|
case 'warn':
|
|
$output = '';
|
|
$formName = $action === 'warn' ? 'wpFilterActionWarn' : 'wpFilterActionDisallow';
|
|
$checkbox =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\CheckboxInputWidget( [
|
|
'name' => $formName,
|
|
// mw-abusefilter-action-checkbox-warn, mw-abusefilter-action-checkbox-disallow
|
|
'id' => "mw-abusefilter-action-checkbox-$action",
|
|
'selected' => $set,
|
|
'classes' => [ 'mw-abusefilter-action-checkbox' ],
|
|
'disabled' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
// abusefilter-edit-action-warn, abusefilter-edit-action-disallow
|
|
'label' => $this->msg( "abusefilter-edit-action-$action" )->text(),
|
|
'align' => 'inline'
|
|
]
|
|
);
|
|
$output .= $checkbox;
|
|
$defaultWarnMsg = $config->get( 'AbuseFilterDefaultWarningMessage' );
|
|
$defaultDisallowMsg = $config->get( 'AbuseFilterDefaultDisallowMessage' );
|
|
|
|
if ( $set && isset( $parameters[0] ) ) {
|
|
$msg = $parameters[0];
|
|
} elseif (
|
|
( $action === 'warn' && isset( $defaultWarnMsg[$filterObj->getGroup()] ) ) ||
|
|
( $action === 'disallow' && isset( $defaultDisallowMsg[$filterObj->getGroup()] ) )
|
|
) {
|
|
$msg = $action === 'warn' ? $defaultWarnMsg[$filterObj->getGroup()] :
|
|
$defaultDisallowMsg[$filterObj->getGroup()];
|
|
} else {
|
|
$msg = $action === 'warn' ? 'abusefilter-warning' : 'abusefilter-disallowed';
|
|
}
|
|
|
|
$fields = [];
|
|
$fields["abusefilter-edit-$action-message"] =
|
|
$this->getExistingSelector( $msg, $readOnly, $action );
|
|
$otherFieldName = $action === 'warn' ? 'wpFilterWarnMessageOther'
|
|
: 'wpFilterDisallowMessageOther';
|
|
|
|
$fields["abusefilter-edit-$action-other-label"] =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\TextInputWidget( [
|
|
'name' => $otherFieldName,
|
|
'value' => $msg,
|
|
// mw-abusefilter-warn-message-other, mw-abusefilter-disallow-message-other
|
|
'id' => "mw-abusefilter-$action-message-other",
|
|
'infusable' => true,
|
|
'readOnly' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => new OOUI\HtmlSnippet(
|
|
// abusefilter-edit-warn-other-label, abusefilter-edit-disallow-other-label
|
|
$this->msg( "abusefilter-edit-$action-other-label" )->parse()
|
|
)
|
|
]
|
|
);
|
|
|
|
$previewButton =
|
|
new OOUI\ButtonInputWidget( [
|
|
// abusefilter-edit-warn-preview, abusefilter-edit-disallow-preview
|
|
'label' => $this->msg( "abusefilter-edit-$action-preview" )->text(),
|
|
// mw-abusefilter-warn-preview-button, mw-abusefilter-disallow-preview-button
|
|
'id' => "mw-abusefilter-$action-preview-button",
|
|
'infusable' => true,
|
|
'flags' => 'progressive'
|
|
]
|
|
);
|
|
|
|
$buttonGroup = $previewButton;
|
|
if ( MediaWikiServices::getInstance()->getPermissionManager()
|
|
->userHasRight( $user, 'editinterface' )
|
|
) {
|
|
$editButton =
|
|
new OOUI\ButtonInputWidget( [
|
|
// abusefilter-edit-warn-edit, abusefilter-edit-disallow-edit
|
|
'label' => $this->msg( "abusefilter-edit-$action-edit" )->text(),
|
|
// mw-abusefilter-warn-edit-button, mw-abusefilter-disallow-edit-button
|
|
'id' => "mw-abusefilter-$action-edit-button"
|
|
]
|
|
);
|
|
$buttonGroup =
|
|
new OOUI\Widget( [
|
|
'content' =>
|
|
new OOUI\HorizontalLayout( [
|
|
'items' => [ $previewButton, $editButton ],
|
|
'classes' => [
|
|
'mw-abusefilter-preview-buttons',
|
|
'mw-abusefilter-javascript-tools'
|
|
]
|
|
] )
|
|
] );
|
|
}
|
|
$previewHolder = Xml::tags(
|
|
'div',
|
|
[
|
|
// mw-abusefilter-warn-preview, mw-abusefilter-disallow-preview
|
|
'id' => "mw-abusefilter-$action-preview",
|
|
'style' => 'display:none'
|
|
],
|
|
''
|
|
);
|
|
$fields["abusefilter-edit-$action-actions"] = $buttonGroup;
|
|
$output .=
|
|
Xml::tags(
|
|
'div',
|
|
// mw-abusefilter-warn-parameters, mw-abusefilter-disallow-parameters
|
|
[ 'id' => "mw-abusefilter-$action-parameters" ],
|
|
new OOUI\FieldsetLayout( [ 'items' => $fields ] )
|
|
) . $previewHolder;
|
|
|
|
return $output;
|
|
case 'tag':
|
|
$tags = $set ? $parameters : [];
|
|
'@phan-var string[] $parameters';
|
|
$output = '';
|
|
|
|
$checkbox =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\CheckboxInputWidget( [
|
|
'name' => 'wpFilterActionTag',
|
|
'id' => 'mw-abusefilter-action-checkbox-tag',
|
|
'selected' => $set,
|
|
'classes' => [ 'mw-abusefilter-action-checkbox' ],
|
|
'disabled' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-action-tag' )->text(),
|
|
'align' => 'inline'
|
|
]
|
|
);
|
|
$output .= $checkbox;
|
|
|
|
$tagConfig = [
|
|
'values' => $tags,
|
|
'label' => $this->msg( 'abusefilter-edit-tag-tag' )->parse(),
|
|
'disabled' => $readOnly
|
|
];
|
|
$this->getOutput()->addJsConfigVars( 'tagConfig', $tagConfig );
|
|
|
|
$hiddenTags =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\MultilineTextInputWidget( [
|
|
'name' => 'wpFilterTags',
|
|
'value' => implode( ',', $tags ),
|
|
'rows' => 5,
|
|
'placeholder' => $this->msg( 'abusefilter-edit-tag-hidden-placeholder' )->text(),
|
|
'infusable' => true,
|
|
'id' => 'mw-abusefilter-hidden-tag-field',
|
|
'readOnly' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => new OOUI\HtmlSnippet(
|
|
$this->msg( 'abusefilter-edit-tag-tag' )->parse()
|
|
),
|
|
'align' => 'top',
|
|
'id' => 'mw-abusefilter-hidden-tag'
|
|
]
|
|
);
|
|
$output .=
|
|
Xml::tags( 'div',
|
|
[ 'id' => 'mw-abusefilter-tag-parameters' ],
|
|
$hiddenTags
|
|
);
|
|
return $output;
|
|
case 'block':
|
|
if ( $set && count( $parameters ) === 3 ) {
|
|
// Both blocktalk and custom block durations available
|
|
list( $blockTalk, $defaultAnonDuration, $defaultUserDuration ) = $parameters;
|
|
} else {
|
|
if ( $set && count( $parameters ) === 1 ) {
|
|
// Only blocktalk available
|
|
// @phan-suppress-next-line PhanTypeArraySuspiciousNullable $parameters is array here
|
|
$blockTalk = $parameters[0];
|
|
}
|
|
if ( $config->get( 'AbuseFilterAnonBlockDuration' ) ) {
|
|
$defaultAnonDuration = $config->get( 'AbuseFilterAnonBlockDuration' );
|
|
} else {
|
|
$defaultAnonDuration = $config->get( 'AbuseFilterBlockDuration' );
|
|
}
|
|
$defaultUserDuration = $config->get( 'AbuseFilterBlockDuration' );
|
|
}
|
|
$suggestedBlocks = SpecialBlock::getSuggestedDurations( null, false );
|
|
$suggestedBlocks = self::normalizeBlocks( $suggestedBlocks );
|
|
|
|
$output = '';
|
|
$checkbox =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\CheckboxInputWidget( [
|
|
'name' => 'wpFilterActionBlock',
|
|
'id' => 'mw-abusefilter-action-checkbox-block',
|
|
'selected' => $set,
|
|
'classes' => [ 'mw-abusefilter-action-checkbox' ],
|
|
'disabled' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-action-block' )->text(),
|
|
'align' => 'inline'
|
|
]
|
|
);
|
|
$output .= $checkbox;
|
|
|
|
$suggestedBlocks = Xml::listDropDownOptionsOoui( $suggestedBlocks );
|
|
|
|
$anonDuration =
|
|
new OOUI\DropdownInputWidget( [
|
|
'name' => 'wpBlockAnonDuration',
|
|
'options' => $suggestedBlocks,
|
|
'value' => $defaultAnonDuration,
|
|
'disabled' => $readOnly
|
|
] );
|
|
|
|
$userDuration =
|
|
new OOUI\DropdownInputWidget( [
|
|
'name' => 'wpBlockUserDuration',
|
|
'options' => $suggestedBlocks,
|
|
'value' => $defaultUserDuration,
|
|
'disabled' => $readOnly
|
|
] );
|
|
|
|
$blockOptions = [];
|
|
if ( $config->get( 'BlockAllowsUTEdit' ) === true ) {
|
|
$talkCheckbox =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\CheckboxInputWidget( [
|
|
'name' => 'wpFilterBlockTalk',
|
|
'id' => 'mw-abusefilter-action-checkbox-blocktalk',
|
|
'selected' => isset( $blockTalk ) && $blockTalk === 'blocktalk',
|
|
'classes' => [ 'mw-abusefilter-action-checkbox' ],
|
|
'disabled' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-action-blocktalk' )->text(),
|
|
'align' => 'left'
|
|
]
|
|
);
|
|
|
|
$blockOptions['abusefilter-edit-block-options'] = $talkCheckbox;
|
|
}
|
|
$blockOptions['abusefilter-edit-block-anon-durations'] =
|
|
new OOUI\FieldLayout(
|
|
$anonDuration,
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-block-anon-durations' )->text()
|
|
]
|
|
);
|
|
$blockOptions['abusefilter-edit-block-user-durations'] =
|
|
new OOUI\FieldLayout(
|
|
$userDuration,
|
|
[
|
|
'label' => $this->msg( 'abusefilter-edit-block-user-durations' )->text()
|
|
]
|
|
);
|
|
|
|
$output .= Xml::tags(
|
|
'div',
|
|
[ 'id' => 'mw-abusefilter-block-parameters' ],
|
|
new OOUI\FieldsetLayout( [ 'items' => $blockOptions ] )
|
|
);
|
|
|
|
return $output;
|
|
|
|
default:
|
|
// Give grep a chance to find the usages:
|
|
// abusefilter-edit-action-disallow,
|
|
// abusefilter-edit-action-blockautopromote,
|
|
// abusefilter-edit-action-degroup,
|
|
// abusefilter-edit-action-rangeblock,
|
|
$message = 'abusefilter-edit-action-' . $action;
|
|
$form_field = 'wpFilterAction' . ucfirst( $action );
|
|
$status = $set;
|
|
|
|
$thisAction =
|
|
new OOUI\FieldLayout(
|
|
new OOUI\CheckboxInputWidget( [
|
|
'name' => $form_field,
|
|
'id' => "mw-abusefilter-action-checkbox-$action",
|
|
'selected' => $status,
|
|
'classes' => [ 'mw-abusefilter-action-checkbox' ],
|
|
'disabled' => $readOnly
|
|
]
|
|
),
|
|
[
|
|
'label' => $this->msg( $message )->text(),
|
|
'align' => 'inline'
|
|
]
|
|
);
|
|
return $thisAction;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @param string $warnMsg
|
|
* @param bool $readOnly
|
|
* @param string $action
|
|
* @return \OOUI\FieldLayout
|
|
*/
|
|
public function getExistingSelector( $warnMsg, $readOnly = false, $action = 'warn' ) {
|
|
if ( $action === 'warn' ) {
|
|
$action = 'warning';
|
|
$formId = 'warn';
|
|
$inputName = 'wpFilterWarnMessage';
|
|
} elseif ( $action === 'disallow' ) {
|
|
$action = 'disallowed';
|
|
$formId = 'disallow';
|
|
$inputName = 'wpFilterDisallowMessage';
|
|
} else {
|
|
throw new MWException( "Unexpected action value $action" );
|
|
}
|
|
|
|
$existingSelector =
|
|
new OOUI\DropdownInputWidget( [
|
|
'name' => $inputName,
|
|
// mw-abusefilter-warn-message-existing, mw-abusefilter-disallow-message-existing
|
|
'id' => "mw-abusefilter-$formId-message-existing",
|
|
// abusefilter-warning, abusefilter-disallowed
|
|
'value' => $warnMsg === "abusefilter-$action" ? "abusefilter-$action" : 'other',
|
|
'infusable' => true
|
|
] );
|
|
|
|
// abusefilter-warning, abusefilter-disallowed
|
|
$options = [ "abusefilter-$action" => "abusefilter-$action" ];
|
|
|
|
if ( $readOnly ) {
|
|
$existingSelector->setDisabled( true );
|
|
} else {
|
|
// Find other messages.
|
|
$dbr = wfGetDB( DB_REPLICA );
|
|
$pageTitlePrefix = "Abusefilter-$action";
|
|
$titles = $dbr->selectFieldValues(
|
|
'page',
|
|
'page_title',
|
|
[
|
|
'page_namespace' => 8,
|
|
'page_title LIKE ' . $dbr->addQuotes( $pageTitlePrefix . '%' )
|
|
],
|
|
__METHOD__
|
|
);
|
|
|
|
$lang = $this->getLanguage();
|
|
foreach ( $titles as $title ) {
|
|
if ( $lang->lcfirst( $title ) === $lang->lcfirst( $warnMsg ) ) {
|
|
$existingSelector->setValue( $lang->lcfirst( $warnMsg ) );
|
|
}
|
|
|
|
if ( $title !== "Abusefilter-$action" ) {
|
|
$options[ $lang->lcfirst( $title ) ] = $lang->lcfirst( $title );
|
|
}
|
|
}
|
|
}
|
|
|
|
// abusefilter-edit-warn-other, abusefilter-edit-disallow-other
|
|
$options[ $this->msg( "abusefilter-edit-$formId-other" )->text() ] = 'other';
|
|
|
|
$options = Xml::listDropDownOptionsOoui( $options );
|
|
$existingSelector->setOptions( $options );
|
|
|
|
$existingSelector =
|
|
new OOUI\FieldLayout(
|
|
$existingSelector,
|
|
[
|
|
// abusefilter-edit-warn-message, abusefilter-edit-disallow-message
|
|
'label' => $this->msg( "abusefilter-edit-$formId-message" )->text()
|
|
]
|
|
);
|
|
|
|
return $existingSelector;
|
|
}
|
|
|
|
/**
|
|
* @todo Maybe we should also check if global values belong to $durations
|
|
* and determine the right point to add them if missing.
|
|
*
|
|
* @param array $durations
|
|
* @return array
|
|
*/
|
|
protected static function normalizeBlocks( array $durations ) {
|
|
global $wgAbuseFilterBlockDuration, $wgAbuseFilterAnonBlockDuration;
|
|
// We need to have same values since it may happen that ipblocklist
|
|
// and one (or both) of the global variables use different wording
|
|
// for the same duration. In such case, when setting the default of
|
|
// the dropdowns it would fail.
|
|
$anonDuration = self::getAbsoluteBlockDuration( $wgAbuseFilterAnonBlockDuration );
|
|
$userDuration = self::getAbsoluteBlockDuration( $wgAbuseFilterBlockDuration );
|
|
foreach ( $durations as &$duration ) {
|
|
$currentDuration = self::getAbsoluteBlockDuration( $duration );
|
|
|
|
if ( $duration !== $wgAbuseFilterBlockDuration &&
|
|
$currentDuration === $userDuration ) {
|
|
$duration = $wgAbuseFilterBlockDuration;
|
|
|
|
} elseif ( $duration !== $wgAbuseFilterAnonBlockDuration &&
|
|
$currentDuration === $anonDuration ) {
|
|
$duration = $wgAbuseFilterAnonBlockDuration;
|
|
}
|
|
}
|
|
|
|
return $durations;
|
|
}
|
|
|
|
/**
|
|
* Converts a string duration to an absolute timestamp, i.e. unrelated to the current
|
|
* time, taking into account infinity durations as well. The second parameter of
|
|
* strtotime is set to 0 in order to convert the duration in seconds (instead of
|
|
* a timestamp), thus making it unaffected by the execution time of the code.
|
|
*
|
|
* @param string $duration
|
|
* @return string|int
|
|
*/
|
|
protected static function getAbsoluteBlockDuration( $duration ) {
|
|
if ( wfIsInfinity( $duration ) ) {
|
|
return 'infinity';
|
|
}
|
|
return strtotime( $duration, 0 );
|
|
}
|
|
|
|
/**
|
|
* Loads filter data from the database by ID.
|
|
* @param int|null $id The filter's ID number, or null for a new filter
|
|
* @return Filter
|
|
* @throws FilterNotFoundException
|
|
*/
|
|
private function loadFilterData( ?int $id ) : Filter {
|
|
if ( $id === null ) {
|
|
return MutableFilter::newDefault();
|
|
}
|
|
|
|
$filterLookup = AbuseFilterServices::getFilterLookup();
|
|
$flags = $this->getRequest()->wasPosted()
|
|
// Load from master to avoid unintended reversions where there's replication lag.
|
|
? FilterLookup::READ_LATEST
|
|
: FilterLookup::READ_NORMAL;
|
|
|
|
return $filterLookup->getFilter( $id, false, $flags );
|
|
}
|
|
|
|
/**
|
|
* Load filter data to show in the edit view from the DB.
|
|
* @param int|null $filter The filter ID being requested or null for a new filter
|
|
* @param int|null $history_id If any, the history ID being requested.
|
|
* @return Filter|null Null if the filter does not exist.
|
|
*/
|
|
private function loadFromDatabase( ?int $filter, $history_id = null ) : ?Filter {
|
|
if ( $history_id ) {
|
|
try {
|
|
return AbuseFilterServices::getFilterLookup()->getFilterVersion( $history_id );
|
|
} catch ( FilterVersionNotFoundException $_ ) {
|
|
return null;
|
|
}
|
|
} else {
|
|
return $this->loadFilterData( $filter );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Load data from the HTTP request. Used for saving the filter, and when the token doesn't match
|
|
* @param int|null $filter
|
|
* @return Filter[]
|
|
*/
|
|
private function loadRequest( ?int $filter ) : array {
|
|
$request = $this->getRequest();
|
|
if ( !$request->wasPosted() ) {
|
|
// Sanity
|
|
throw new BadMethodCallException( __METHOD__ . ' called without the request being POSTed.' );
|
|
}
|
|
|
|
$origFilter = $this->loadFilterData( $filter );
|
|
|
|
$newFilter = $origFilter instanceof MutableFilter
|
|
? clone $origFilter
|
|
: MutableFilter::newFromParentFilter( $origFilter );
|
|
|
|
if ( $filter !== null ) {
|
|
// Unchangeable values
|
|
// @phan-suppress-next-line PhanTypeMismatchArgumentNullable
|
|
$newFilter->setThrottled( $origFilter->isThrottled() );
|
|
// @phan-suppress-next-line PhanTypeMismatchArgumentNullable
|
|
$newFilter->setHitCount( $origFilter->getHitCount() );
|
|
// These are needed if the save fails and the filter is not new
|
|
$newFilter->setID( $origFilter->getID() );
|
|
$newFilter->setUserID( $origFilter->getUserID() );
|
|
$newFilter->setUserName( $origFilter->getUserName() );
|
|
$newFilter->setTimestamp( $origFilter->getTimestamp() );
|
|
}
|
|
|
|
$newFilter->setName( trim( $request->getVal( 'wpFilterDescription' ) ) );
|
|
$newFilter->setRules( trim( $request->getVal( 'wpFilterRules' ) ) );
|
|
$newFilter->setComments( trim( $request->getVal( 'wpFilterNotes' ) ) );
|
|
|
|
$newFilter->setGroup( $request->getVal( 'wpFilterGroup', 'default' ) );
|
|
|
|
$newFilter->setDeleted( $request->getCheck( 'wpFilterDeleted' ) );
|
|
$newFilter->setEnabled( $request->getCheck( 'wpFilterEnabled' ) );
|
|
$newFilter->setHidden( $request->getCheck( 'wpFilterHidden' ) );
|
|
$newFilter->setGlobal( $request->getCheck( 'wpFilterGlobal' )
|
|
&& $this->getConfig()->get( 'AbuseFilterIsCentral' ) );
|
|
|
|
$actions = $this->loadActions();
|
|
|
|
$newFilter->setActions( $actions );
|
|
|
|
return [ $newFilter, $origFilter ];
|
|
}
|
|
|
|
/**
|
|
* @return Filter|null
|
|
*/
|
|
private function loadImportRequest() : ?Filter {
|
|
$validGroups = $this->getConfig()->get( 'AbuseFilterValidGroups' );
|
|
$globalFiltersEnabled = $this->getConfig()->get( 'AbuseFilterIsCentral' );
|
|
$request = $this->getRequest();
|
|
if ( !$request->wasPosted() ) {
|
|
// Sanity
|
|
throw new BadMethodCallException( __METHOD__ . ' called without the request being POSTed.' );
|
|
}
|
|
|
|
$importData = FormatJson::decode( $request->getVal( 'wpImportText' ) );
|
|
if ( !$this->isValidImportData( $importData ) ) {
|
|
return null;
|
|
}
|
|
|
|
$importRow = $importData->row;
|
|
$actions = wfObjectToArray( $importData->actions );
|
|
|
|
return new MutableFilter(
|
|
new Specs(
|
|
$importRow->af_pattern,
|
|
$importRow->af_comments,
|
|
$importRow->af_public_comments,
|
|
array_keys( $actions ),
|
|
// Keep the group only if it exists on this wiki
|
|
in_array( $importRow->af_group, $validGroups, true ) ? $importRow->af_group : 'default'
|
|
),
|
|
new Flags(
|
|
(bool)$importRow->af_enabled,
|
|
(bool)$importRow->af_deleted,
|
|
(bool)$importRow->af_hidden,
|
|
// And also make it global only if global filters are enabled here
|
|
$importRow->af_global && $globalFiltersEnabled
|
|
),
|
|
$actions,
|
|
new LastEditInfo(
|
|
0,
|
|
'',
|
|
''
|
|
)
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @return array[]
|
|
*/
|
|
private function loadActions() : array {
|
|
$request = $this->getRequest();
|
|
$actions = [];
|
|
foreach ( array_filter( $this->getConfig()->get( 'AbuseFilterActions' ) ) as $action => $_ ) {
|
|
// Check if it's set
|
|
$enabled = $request->getCheck( 'wpFilterAction' . ucfirst( $action ) );
|
|
|
|
if ( $enabled ) {
|
|
$parameters = [];
|
|
|
|
if ( $action === 'throttle' ) {
|
|
// We need to load the parameters
|
|
$throttleCount = $request->getIntOrNull( 'wpFilterThrottleCount' );
|
|
$throttlePeriod = $request->getIntOrNull( 'wpFilterThrottlePeriod' );
|
|
// First explode with \n, which is the delimiter used in the textarea
|
|
$rawGroups = explode( "\n", $request->getText( 'wpFilterThrottleGroups' ) );
|
|
// Trim any space, both as an actual group and inside subgroups
|
|
$throttleGroups = [];
|
|
foreach ( $rawGroups as $group ) {
|
|
if ( strpos( $group, ',' ) !== false ) {
|
|
$subGroups = explode( ',', $group );
|
|
$throttleGroups[] = implode( ',', array_map( 'trim', $subGroups ) );
|
|
} elseif ( trim( $group ) !== '' ) {
|
|
$throttleGroups[] = trim( $group );
|
|
}
|
|
}
|
|
|
|
$parameters[0] = $this->filter;
|
|
$parameters[1] = "$throttleCount,$throttlePeriod";
|
|
$parameters = array_merge( $parameters, $throttleGroups );
|
|
} elseif ( $action === 'warn' ) {
|
|
$specMsg = $request->getVal( 'wpFilterWarnMessage' );
|
|
|
|
if ( $specMsg === 'other' ) {
|
|
$specMsg = $request->getVal( 'wpFilterWarnMessageOther' );
|
|
}
|
|
|
|
$parameters[0] = $specMsg;
|
|
} elseif ( $action === 'block' ) {
|
|
$parameters[0] = $request->getCheck( 'wpFilterBlockTalk' ) ?
|
|
'blocktalk' : 'noTalkBlockSet';
|
|
$parameters[1] = $request->getVal( 'wpBlockAnonDuration' );
|
|
$parameters[2] = $request->getVal( 'wpBlockUserDuration' );
|
|
} elseif ( $action === 'disallow' ) {
|
|
$specMsg = $request->getVal( 'wpFilterDisallowMessage' );
|
|
|
|
if ( $specMsg === 'other' ) {
|
|
$specMsg = $request->getVal( 'wpFilterDisallowMessageOther' );
|
|
}
|
|
|
|
$parameters[0] = $specMsg;
|
|
} elseif ( $action === 'tag' ) {
|
|
$parameters = explode( ',', trim( $request->getText( 'wpFilterTags' ) ) );
|
|
if ( $parameters === [ '' ] ) {
|
|
// Since it's not possible to manually add an empty tag, this only happens
|
|
// if the form is submitted without touching the tag input field.
|
|
// We pass an empty array so that the widget won't show an empty tag in the topbar
|
|
$parameters = [];
|
|
}
|
|
}
|
|
|
|
$actions[$action] = $parameters;
|
|
}
|
|
}
|
|
return $actions;
|
|
}
|
|
|
|
/**
|
|
* Exports the default warning and disallow messages to a JS variable
|
|
*/
|
|
protected function exposeMessages() {
|
|
$this->getOutput()->addJsConfigVars(
|
|
'wgAbuseFilterDefaultWarningMessage',
|
|
$this->getConfig()->get( 'AbuseFilterDefaultWarningMessage' )
|
|
);
|
|
$this->getOutput()->addJsConfigVars(
|
|
'wgAbuseFilterDefaultDisallowMessage',
|
|
$this->getConfig()->get( 'AbuseFilterDefaultDisallowMessage' )
|
|
);
|
|
}
|
|
|
|
/**
|
|
* Perform basic validation on the JSON-decoded import data. This doesn't check if parameters
|
|
* are valid etc., but only if the shape of the object is right.
|
|
* @todo This should live in ViewImport, but that's nontrivial due to form action
|
|
*
|
|
* @param mixed $data Already JSON-decoded
|
|
* @return bool
|
|
*/
|
|
private function isValidImportData( $data ) {
|
|
global $wgAbuseFilterActions;
|
|
|
|
if ( !is_object( $data ) ) {
|
|
return false;
|
|
}
|
|
|
|
$arr = get_object_vars( $data );
|
|
|
|
$expectedKeys = [ 'row' => true, 'actions' => true ];
|
|
if ( count( $arr ) !== count( $expectedKeys ) || array_diff_key( $arr, $expectedKeys ) ) {
|
|
return false;
|
|
}
|
|
|
|
if ( !is_object( $arr['row'] ) || !( is_object( $arr['actions'] ) || $arr['actions'] === [] ) ) {
|
|
return false;
|
|
}
|
|
|
|
foreach ( $arr['actions'] as $action => $params ) {
|
|
if ( !array_key_exists( $action, $wgAbuseFilterActions ) || !is_array( $params ) ) {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
$completeRow = (object)array_merge(
|
|
get_object_vars( $arr['row'] ),
|
|
array_fill_keys( self::EXPORT_EXCLUDED_PROPS, 1 )
|
|
);
|
|
if ( !AbuseFilter::isFullAbuseFilterRow( $completeRow ) ) {
|
|
return false;
|
|
}
|
|
|
|
return true;
|
|
}
|
|
}
|