mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 02:03:53 +00:00
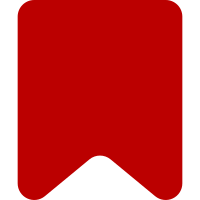
Adding PHPdocs to every class members, in every file. This patch only touches comments, and moved properties on their own lines. Note that some of these properties would need to be moved, somehow changed, or just removed (either because they're old, unused leftovers, or just because we can move them to local scope), but I wanted to keep this patch doc-only. Change-Id: I9fe701445bea8f09d82783789ff1ec537ac6704b
75 lines
1.8 KiB
PHP
75 lines
1.8 KiB
PHP
<?php
|
|
/**
|
|
* Abuse filter parser.
|
|
* Copyright © Victor Vasiliev, 2008.
|
|
* Based on ideas by Andrew Garrett
|
|
* Distributed under GNU GPL v2 terms.
|
|
*
|
|
* Types of token:
|
|
* * T_NONE - special-purpose token
|
|
* * T_BRACE - ( or )
|
|
* * T_COMMA - ,
|
|
* * T_OP - operator like + or ^
|
|
* * T_NUMBER - number
|
|
* * T_STRING - string, in "" or ''
|
|
* * T_KEYWORD - keyword
|
|
* * T_ID - identifier
|
|
* * T_STATEMENT_SEPARATOR - ;
|
|
* * T_SQUARE_BRACKETS - [ or ]
|
|
*
|
|
* Levels of parsing:
|
|
* * Entry - catches unexpected characters
|
|
* * Semicolon - ;
|
|
* * Set - :=
|
|
* * Conditionals (IF) - if-then-else-end, cond ? a :b
|
|
* * BoolOps (BO) - &, |, ^
|
|
* * CompOps (CO) - ==, !=, ===, !==, >, <, >=, <=
|
|
* * SumRel (SR) - +, -
|
|
* * MulRel (MR) - *, /, %
|
|
* * Pow (P) - **
|
|
* * BoolNeg (BN) - ! operation
|
|
* * SpecialOperators (SO) - in and like
|
|
* * Unarys (U) - plus and minus in cases like -5 or -(2 * +2)
|
|
* * ArrayElement (AE) - array[number]
|
|
* * Braces (B) - ( and )
|
|
* * Functions (F)
|
|
* * Atom (A) - return value
|
|
*/
|
|
class AFPToken {
|
|
const TNONE = 'T_NONE';
|
|
const TID = 'T_ID';
|
|
const TKEYWORD = 'T_KEYWORD';
|
|
const TSTRING = 'T_STRING';
|
|
const TINT = 'T_INT';
|
|
const TFLOAT = 'T_FLOAT';
|
|
const TOP = 'T_OP';
|
|
const TBRACE = 'T_BRACE';
|
|
const TSQUAREBRACKET = 'T_SQUARE_BRACKET';
|
|
const TCOMMA = 'T_COMMA';
|
|
const TSTATEMENTSEPARATOR = 'T_STATEMENT_SEPARATOR';
|
|
|
|
/**
|
|
* @var string One of the T* constant from this class
|
|
*/
|
|
public $type;
|
|
/**
|
|
* @var mixed|null The actual value of the token
|
|
*/
|
|
public $value;
|
|
/**
|
|
* @var int The code offset where this token is found
|
|
*/
|
|
public $pos;
|
|
|
|
/**
|
|
* @param string $type
|
|
* @param mixed|null $value
|
|
* @param int $pos
|
|
*/
|
|
public function __construct( $type = self::TNONE, $value = null, $pos = 0 ) {
|
|
$this->type = $type;
|
|
$this->value = $value;
|
|
$this->pos = $pos;
|
|
}
|
|
}
|