mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 02:03:53 +00:00
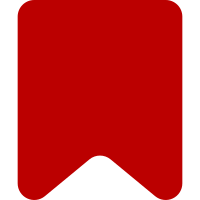
There are lots of cases where we can inject a User object without additional efforts. Now $wgUser is only used inside AFComputedVariable, which is a little bit harder to handle because some instances of that class are serialized in the DB, and thus we cannot easily change the constructor until T213006 is resolved. This partly copies what Ia474f02dfeee8c7d067ee7e555c08cbfef08f6a6 tried to do, but adopting a different approach for various can*() methods: they're now static methods in the AbuseFilter class, so future callers don't need to instantiate an AbuseFilterView class. This also allows to re-use those methods in an API module for editing filters (T213037). Bug: T213037 Bug: T159299 Change-Id: I22743557e162fd23b3b4e52951a649d8c21109c8
89 lines
1.5 KiB
PHP
89 lines
1.5 KiB
PHP
<?php
|
|
|
|
use Wikimedia\Rdbms\IResultWrapper;
|
|
|
|
class AbuseLogPager extends ReverseChronologicalPager {
|
|
/**
|
|
* @var SpecialAbuseLog
|
|
*/
|
|
public $mForm;
|
|
|
|
/**
|
|
* @var array
|
|
*/
|
|
public $mConds;
|
|
|
|
/**
|
|
* @param SpecialAbuseLog $form
|
|
* @param array $conds
|
|
*/
|
|
public function __construct( SpecialAbuseLog $form, $conds = [] ) {
|
|
$this->mForm = $form;
|
|
$this->mConds = $conds;
|
|
parent::__construct();
|
|
}
|
|
|
|
/**
|
|
* @param object $row
|
|
* @return string
|
|
*/
|
|
public function formatRow( $row ) {
|
|
return $this->mForm->formatRow( $row );
|
|
}
|
|
|
|
/**
|
|
* @return array
|
|
*/
|
|
public function getQueryInfo() {
|
|
$conds = $this->mConds;
|
|
|
|
$info = [
|
|
'tables' => [ 'abuse_filter_log', 'abuse_filter' ],
|
|
'fields' => '*',
|
|
'conds' => $conds,
|
|
'join_conds' =>
|
|
[ 'abuse_filter' =>
|
|
[
|
|
'LEFT JOIN',
|
|
'af_id=afl_filter',
|
|
],
|
|
],
|
|
];
|
|
|
|
if ( !$this->mForm->canSeeHidden( $this->getUser() ) ) {
|
|
$info['conds']['afl_deleted'] = 0;
|
|
}
|
|
|
|
return $info;
|
|
}
|
|
|
|
/**
|
|
* @param IResultWrapper $result
|
|
*/
|
|
protected function preprocessResults( $result ) {
|
|
if ( $this->getNumRows() === 0 ) {
|
|
return;
|
|
}
|
|
|
|
$lb = new LinkBatch();
|
|
$lb->setCaller( __METHOD__ );
|
|
foreach ( $result as $row ) {
|
|
// Only for local wiki results
|
|
if ( !$row->afl_wiki ) {
|
|
$lb->add( $row->afl_namespace, $row->afl_title );
|
|
$lb->add( NS_USER, $row->afl_user );
|
|
$lb->add( NS_USER_TALK, $row->afl_user_text );
|
|
}
|
|
}
|
|
$lb->execute();
|
|
$result->seek( 0 );
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
*/
|
|
public function getIndexField() {
|
|
return 'afl_timestamp';
|
|
}
|
|
}
|