mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-09-24 10:48:17 +00:00
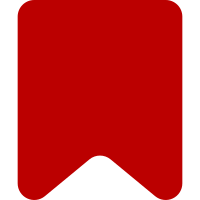
ParserStatus is now more lightweight, and doesn't know about "result" and "from cache". Instead, it has an isValid() method which is merely a shorthand for checking whether getException() is null. Introduce a child class, RuleCheckerStatus, which knows about result and cache and can be (un)serialized. This removes the ambiguity of the $result field, and helps the transition to a new RuleChecker class. Change-Id: I0dac7ab4febbfdabe72596631db630411d967ab5
82 lines
1.9 KiB
PHP
82 lines
1.9 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter\Parser;
|
|
|
|
use MediaWiki\Extension\AbuseFilter\Parser\Exception\ExceptionBase;
|
|
use MediaWiki\Extension\AbuseFilter\Parser\Exception\UserVisibleWarning;
|
|
|
|
class RuleCheckerStatus extends ParserStatus {
|
|
/** @var bool */
|
|
private $result;
|
|
/** @var bool */
|
|
private $warmCache;
|
|
|
|
/**
|
|
* @param bool $result Whether the rule matched
|
|
* @param bool $warmCache Whether we retrieved the AST from cache
|
|
* @param ExceptionBase|null $excep An exception thrown while parsing, or null if it parsed correctly
|
|
* @param UserVisibleWarning[] $warnings
|
|
* @param int $condsUsed
|
|
*/
|
|
public function __construct(
|
|
bool $result,
|
|
bool $warmCache,
|
|
?ExceptionBase $excep,
|
|
array $warnings,
|
|
int $condsUsed
|
|
) {
|
|
parent::__construct( $excep, $warnings, $condsUsed );
|
|
$this->result = $result;
|
|
$this->warmCache = $warmCache;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getResult(): bool {
|
|
return $this->result;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getWarmCache(): bool {
|
|
return $this->warmCache;
|
|
}
|
|
|
|
/**
|
|
* Serialize data for edit stash
|
|
* @return array
|
|
*/
|
|
public function toArray(): array {
|
|
return [
|
|
'result' => $this->result,
|
|
'warmCache' => $this->warmCache,
|
|
'exception' => $this->excep ? $this->excep->toArray() : null,
|
|
'warnings' => array_map(
|
|
static function ( $warn ) {
|
|
return $warn->toArray();
|
|
},
|
|
$this->warnings
|
|
),
|
|
'condsUsed' => $this->condsUsed,
|
|
];
|
|
}
|
|
|
|
/**
|
|
* Deserialize data from edit stash
|
|
* @param array $value
|
|
* @return self
|
|
*/
|
|
public static function fromArray( array $value ): self {
|
|
$excClass = $value['exception']['class'] ?? null;
|
|
return new self(
|
|
$value['result'],
|
|
$value['warmCache'],
|
|
$excClass !== null ? call_user_func( [ $excClass, 'fromArray' ], $value['exception'] ) : null,
|
|
array_map( [ UserVisibleWarning::class, 'fromArray' ], $value['warnings'] ),
|
|
$value['condsUsed']
|
|
);
|
|
}
|
|
}
|