mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-13 17:27:20 +00:00
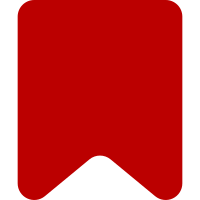
Some exposed variables (eg. `user_ip`) used in filters are sensitive and need to only be available to restricted groups of users. Back-end changes: - Add `AbuseFilterProtectedVariables` which defines what variables are protected by the new right `abusefilter-access-protected-vars` - Add the concept of a `protected` variable, the use of which will denote the entire filter as protected via a flag on `af_hidden` New UX features: - Display changes to the protected status of filters on history and diff pages - Check for protected variables and the right to see them in filter validation and don't allow a filter to be saved if it uses a variable that the user doesn't have access to - Check for the right to view protected variables before allowing access and edits to existing filters that use them Bug: T364465 Bug: T363906 Change-Id: I828bbb4015e87040f69a8e10c7888273c4f24dd3
90 lines
2.9 KiB
PHP
90 lines
2.9 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter\Pager;
|
|
|
|
use MediaWiki\Extension\AbuseFilter\AbuseFilterPermissionManager;
|
|
use MediaWiki\Extension\AbuseFilter\CentralDBManager;
|
|
use MediaWiki\Extension\AbuseFilter\FilterUtils;
|
|
use MediaWiki\Extension\AbuseFilter\SpecsFormatter;
|
|
use MediaWiki\Extension\AbuseFilter\View\AbuseFilterViewList;
|
|
use MediaWiki\Linker\LinkRenderer;
|
|
|
|
/**
|
|
* Class to build paginated filter list for wikis using global abuse filters
|
|
*/
|
|
class GlobalAbuseFilterPager extends AbuseFilterPager {
|
|
|
|
/**
|
|
* @param AbuseFilterViewList $page
|
|
* @param LinkRenderer $linkRenderer
|
|
* @param AbuseFilterPermissionManager $afPermManager
|
|
* @param SpecsFormatter $specsFormatter
|
|
* @param CentralDBManager $centralDBManager
|
|
* @param array $conds
|
|
*/
|
|
public function __construct(
|
|
AbuseFilterViewList $page,
|
|
LinkRenderer $linkRenderer,
|
|
AbuseFilterPermissionManager $afPermManager,
|
|
SpecsFormatter $specsFormatter,
|
|
CentralDBManager $centralDBManager,
|
|
array $conds
|
|
) {
|
|
// Set database before parent constructor to avoid setting it there
|
|
$this->mDb = $centralDBManager->getConnection( DB_REPLICA );
|
|
parent::__construct( $page, $linkRenderer, null, $afPermManager, $specsFormatter, $conds, null, null );
|
|
}
|
|
|
|
/**
|
|
* @param string $name
|
|
* @param string|null $value
|
|
* @return string
|
|
*/
|
|
public function formatValue( $name, $value ) {
|
|
$lang = $this->getLanguage();
|
|
$row = $this->mCurrentRow;
|
|
|
|
switch ( $name ) {
|
|
case 'af_id':
|
|
return $lang->formatNum( intval( $value ) );
|
|
case 'af_public_comments':
|
|
return $this->getOutput()->parseInlineAsInterface( $value );
|
|
case 'af_enabled':
|
|
$statuses = [];
|
|
if ( $row->af_deleted ) {
|
|
$statuses[] = $this->msg( 'abusefilter-deleted' )->parse();
|
|
} elseif ( $row->af_enabled ) {
|
|
$statuses[] = $this->msg( 'abusefilter-enabled' )->parse();
|
|
} else {
|
|
$statuses[] = $this->msg( 'abusefilter-disabled' )->parse();
|
|
}
|
|
if ( $row->af_global ) {
|
|
$statuses[] = $this->msg( 'abusefilter-status-global' )->parse();
|
|
}
|
|
|
|
return $lang->commaList( $statuses );
|
|
case 'af_hit_count':
|
|
// If the rule is hidden or protected, don't show it, even to privileged local admins
|
|
if ( FilterUtils::isHidden( $row->af_hidden ) || FilterUtils::isProtected( $row->af_hidden ) ) {
|
|
return '';
|
|
}
|
|
return $this->msg( 'abusefilter-hitcount' )->numParams( $value )->parse();
|
|
case 'af_timestamp':
|
|
$user = $this->getUser();
|
|
return $this->msg(
|
|
'abusefilter-edit-lastmod-text',
|
|
$lang->userTimeAndDate( $value, $user ),
|
|
$row->af_user_text,
|
|
$lang->userDate( $value, $user ),
|
|
$lang->userTime( $value, $user ),
|
|
$row->af_user_text
|
|
)->parse();
|
|
case 'af_group':
|
|
// If this is global, local name probably doesn't exist, but try
|
|
return $this->specsFormatter->nameGroup( $value );
|
|
default:
|
|
return parent::formatValue( $name, $value );
|
|
}
|
|
}
|
|
}
|