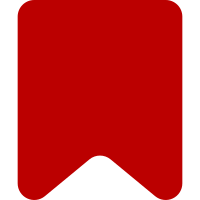
Skip running the generator on interface messages and if a description has already been set. This resolves some annoyances ranging from performance (relevant if the algorithm becomes more expensive to run) to multiple description meta tags being spawned. This is part of my RemexHtml patch chain, which I've split up to avoid having a single commit alter the majority of the codebase. If it ends up being rejected, I can rebase this change to rid of dependencies on the rest of the chain. Depends-On: I97fd065c9554837747021ba9fff26005e33270f4 Change-Id: I585f2c0046571310aad67f3ba148c4f22aaae49f
117 lines
3 KiB
PHP
117 lines
3 KiB
PHP
<?php
|
||
|
||
namespace MediaWiki\Extension\Description2;
|
||
|
||
use Config;
|
||
use ConfigFactory;
|
||
use OutputPage;
|
||
use Parser;
|
||
use ParserOutput;
|
||
|
||
/**
|
||
* Description2 – Adds meaningful description <meta> tag to MW pages and into the parser output
|
||
*
|
||
* @file
|
||
* @ingroup Extensions
|
||
* @author Daniel Friesen (http://danf.ca/mw/)
|
||
* @copyright Copyright 2010 – Daniel Friesen
|
||
* @license GPL-2.0-or-later
|
||
* @link https://www.mediawiki.org/wiki/Extension:Description2 Documentation
|
||
*/
|
||
|
||
class Hooks implements
|
||
\MediaWiki\Hook\ParserAfterTidyHook,
|
||
\MediaWiki\Hook\ParserFirstCallInitHook,
|
||
\MediaWiki\Hook\OutputPageParserOutputHook
|
||
{
|
||
|
||
/** @var Config */
|
||
private Config $config;
|
||
|
||
/** @var DescriptionProvider */
|
||
private DescriptionProvider $descriptionProvider;
|
||
|
||
/**
|
||
* @param ConfigFactory $configFactory
|
||
*/
|
||
public function __construct(
|
||
ConfigFactory $configFactory,
|
||
DescriptionProvider $descriptionProvider
|
||
) {
|
||
$this->config = $configFactory->makeConfig( 'Description2' );
|
||
$this->descriptionProvider = $descriptionProvider;
|
||
}
|
||
|
||
/**
|
||
* @link https://www.mediawiki.org/wiki/Manual:Hooks/ParserAfterTidy
|
||
* @param Parser $parser The parser.
|
||
* @param string &$text The page text.
|
||
* @return bool
|
||
*/
|
||
public function onParserAfterTidy( $parser, &$text ) {
|
||
$parserOutput = $parser->getOutput();
|
||
|
||
// Avoid running the algorithm on interface messages which may waste time
|
||
if ( $parser->getOptions()->getInterfaceMessage() ) {
|
||
return true;
|
||
}
|
||
|
||
// Avoid running the algorithm multiple times if we already have determined the description. This may happen
|
||
// on file pages.
|
||
if ( method_exists( $parserOutput, 'getPageProperty' ) ) {
|
||
// MW 1.38+
|
||
$description = $parserOutput->getPageProperty( 'description' );
|
||
} else {
|
||
$description = $parserOutput->getProperty( 'description' );
|
||
}
|
||
if ( $description ) {
|
||
return true;
|
||
}
|
||
|
||
$desc = $this->descriptionProvider->derive( $text );
|
||
|
||
if ( $desc ) {
|
||
Description2::setDescription( $parser, $desc );
|
||
}
|
||
|
||
return true;
|
||
}
|
||
|
||
/**
|
||
* @param Parser $parser The parser.
|
||
* @return bool
|
||
*/
|
||
public function onParserFirstCallInit( $parser ) {
|
||
if ( !$this->config->get( 'EnableMetaDescriptionFunctions' ) ) {
|
||
// Functions and tags are disabled
|
||
return true;
|
||
}
|
||
$parser->setFunctionHook(
|
||
'description2',
|
||
[ Description2::class, 'parserFunctionCallback' ],
|
||
Parser::SFH_OBJECT_ARGS
|
||
);
|
||
return true;
|
||
}
|
||
|
||
/**
|
||
* @param OutputPage $out The output page to add the meta element to.
|
||
* @param ParserOutput $parserOutput The parser output to get the description from.
|
||
*/
|
||
public function onOutputPageParserOutput( $out, $parserOutput ): void {
|
||
// Export the description from the main parser output into the OutputPage
|
||
if ( method_exists( $parserOutput, 'getPageProperty' ) ) {
|
||
// MW 1.38+
|
||
$description = $parserOutput->getPageProperty( 'description' );
|
||
} else {
|
||
$description = $parserOutput->getProperty( 'description' );
|
||
if ( $description === false ) {
|
||
$description = null;
|
||
}
|
||
}
|
||
if ( $description !== null ) {
|
||
$out->addMeta( 'description', $description );
|
||
}
|
||
}
|
||
}
|