mirror of
https://github.com/StarCitizenTools/mediawiki-skins-Citizen.git
synced 2024-11-17 11:24:40 +00:00
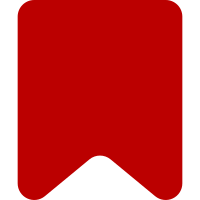
* feat: rewrite search module (WIP) There are some caveats because it is a WIP - Messages are not i18n yet - Missing placeholder suggestion thumbnail - Only REST mode works - Missing greeting message when there is no search query - Code might look like a mess (I learned JS not long ago) * refactor: remove old search module * feat: clean up search suggestion styles * feat: hide overflow for suggestion text * feat: add action API and various cleanup * feat: re-add abort controller * feat: add message support and tweaks * feat: use virtual config instead of ResourceLoader hook * fix: missing comma in const definition * feat: add ARIA attributes
56 lines
1.3 KiB
JavaScript
56 lines
1.3 KiB
JavaScript
/**
|
|
* @typedef {Object} Results
|
|
* @property {string} id The page ID of the page
|
|
* @property {string} title The title of the page.
|
|
* @property {string} description The description of the page.
|
|
* @property {string} thumbnail The url of the thumbnail of the page.
|
|
*
|
|
* @global
|
|
*/
|
|
|
|
const gatewayConfig = require( '../config.json' ).wgCitizenSearchGateway;
|
|
|
|
/**
|
|
* Setup the gateway based on wiki configuration
|
|
*
|
|
* @return {module}
|
|
*/
|
|
function getGateway() {
|
|
switch ( gatewayConfig ) {
|
|
case 'mwActionApi':
|
|
return require( './mwActionApi.js' );
|
|
case 'mwRestApi':
|
|
return require( './mwRestApi.js' );
|
|
default:
|
|
throw new Error( 'Unknown search gateway' );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Fetch suggestion from gateway and return the results object
|
|
*
|
|
* @param {string} searchQuery
|
|
* @param {AbortController} controller
|
|
* @return {Object} Results
|
|
*/
|
|
async function getResults( searchQuery, controller ) {
|
|
const gateway = getGateway();
|
|
|
|
const signal = controller.signal;
|
|
|
|
/* eslint-disable-next-line compat/compat */
|
|
const response = await fetch( gateway.getUrl( searchQuery ), { signal } );
|
|
|
|
if ( !response.ok ) {
|
|
const message = 'Uh oh, a wild error appears! ' + response.status;
|
|
throw new Error( message );
|
|
}
|
|
|
|
const data = await response.json();
|
|
return gateway.convertDataToResults( data );
|
|
}
|
|
|
|
module.exports = {
|
|
getResults: getResults
|
|
};
|