mirror of
https://github.com/StarCitizenTools/mediawiki-skins-Citizen.git
synced 2024-12-04 18:59:03 +00:00
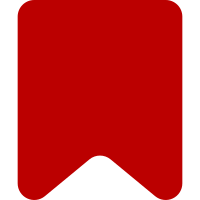
Dropdown + Menu component are already doing the same thing. We can just extend those and drop all the custom handling
52 lines
1.6 KiB
JavaScript
52 lines
1.6 KiB
JavaScript
/**
|
|
* Clientprefs names theme differently from Citizen, we will need to translate it
|
|
* TODO: Migrate to clientprefs fully on MW 1.43
|
|
*/
|
|
const CLIENTPREFS_THEME_MAP = {
|
|
auto: 'os',
|
|
light: 'day',
|
|
dark: 'night'
|
|
};
|
|
|
|
const clientPrefs = require( './clientPrefs.polyfill.js' )();
|
|
|
|
/**
|
|
* Load client preferences based on the existence of 'citizen-preferences__card' element.
|
|
*/
|
|
function loadClientPreferences() {
|
|
const clientPreferenceId = 'citizen-preferences-content';
|
|
const clientPreferenceExists = document.getElementById( clientPreferenceId ) !== null;
|
|
if ( clientPreferenceExists ) {
|
|
const clientPreferences = require( /** @type {string} */( './clientPreferences.js' ) );
|
|
const clientPreferenceConfig = ( require( './clientPreferences.json' ) );
|
|
|
|
clientPreferenceConfig[ 'skin-theme' ].callback = () => {
|
|
const LEGACY_THEME_CLASSES = [
|
|
'skin-citizen-auto',
|
|
'skin-citizen-light',
|
|
'skin-citizen-dark'
|
|
];
|
|
const legacyThemeKey = Object.keys( CLIENTPREFS_THEME_MAP ).find( ( key ) => CLIENTPREFS_THEME_MAP[ key ] === clientPrefs.get( 'skin-theme' ) );
|
|
document.documentElement.classList.remove( ...LEGACY_THEME_CLASSES );
|
|
document.documentElement.classList.add( `skin-citizen-${ legacyThemeKey }` );
|
|
};
|
|
|
|
clientPreferences.render( `#${ clientPreferenceId }`, clientPreferenceConfig );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Set up the listen for preferences button
|
|
*
|
|
* @return {void}
|
|
*/
|
|
function listenForButtonClick() {
|
|
const details = document.getElementById( 'citizen-preferences-details' );
|
|
if ( !details ) {
|
|
return;
|
|
}
|
|
details.addEventListener( 'click', loadClientPreferences, { once: true } );
|
|
}
|
|
|
|
listenForButtonClick();
|