mirror of
https://github.com/StarCitizenTools/mediawiki-skins-Citizen.git
synced 2024-09-23 10:19:43 +00:00
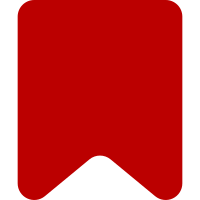
- Refactor existing template data-related partials into CitizenComponent components - Re-implement user menu header as UserInfo - Add description text for anon and temp user in UserMenu --------- Co-authored-by: github-actions <github-actions@users.noreply.github.com>
81 lines
1.8 KiB
PHP
81 lines
1.8 KiB
PHP
<?php
|
|
|
|
declare( strict_types=1 );
|
|
|
|
namespace MediaWiki\Skins\Citizen\Components;
|
|
|
|
/**
|
|
* TODO: Update to new classes when we move to MW 1.43
|
|
* - MediaWiki\Html\Html
|
|
* - MediaWiki\Linker\Linker
|
|
*/
|
|
use Html;
|
|
use Linker;
|
|
use MessageLocalizer;
|
|
|
|
/**
|
|
* CitizenComponentLink component
|
|
*/
|
|
class CitizenComponentLink implements CitizenComponent {
|
|
/** @var MessageLocalizer */
|
|
private $localizer;
|
|
/** @var string */
|
|
private $icon;
|
|
/** @var string */
|
|
private $href;
|
|
/** @var string */
|
|
private $text;
|
|
/** @var string */
|
|
private $accessKeyHint;
|
|
|
|
/**
|
|
* @param string $href
|
|
* @param string $text
|
|
* @param null|string $icon
|
|
* @param null|MessageLocalizer $localizer for generation of tooltip and access keys
|
|
* @param null|string $accessKeyHint will be used to derive HTML attributes such as title, accesskey
|
|
* and aria-label ("$accessKeyHint-label")
|
|
*/
|
|
public function __construct( string $href, string $text, $icon = null, $localizer = null, $accessKeyHint = null ) {
|
|
$this->href = $href;
|
|
$this->text = $text;
|
|
$this->icon = $icon;
|
|
$this->localizer = $localizer;
|
|
$this->accessKeyHint = $accessKeyHint;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getTemplateData(): array {
|
|
$localizer = $this->localizer;
|
|
$accessKeyHint = $this->accessKeyHint;
|
|
$additionalAttributes = [];
|
|
if ( $localizer ) {
|
|
$msg = $localizer->msg( $accessKeyHint . '-label' );
|
|
if ( $msg->exists() ) {
|
|
$additionalAttributes[ 'aria-label' ] = $msg->text();
|
|
}
|
|
}
|
|
return [
|
|
'href' => $this->href,
|
|
'icon' => $this->icon,
|
|
'text' => $this->text,
|
|
'array-attributes' => [
|
|
[
|
|
'key' => 'href',
|
|
'value' => $this->href
|
|
]
|
|
],
|
|
'html-attributes' => $localizer && $accessKeyHint ? Html::expandAttributes(
|
|
Linker::tooltipAndAccesskeyAttribs(
|
|
$accessKeyHint,
|
|
[],
|
|
[],
|
|
$localizer
|
|
) + $additionalAttributes
|
|
) : '',
|
|
];
|
|
}
|
|
}
|